五子棋C++评估函数怎么写,写个例子
时间: 2024-09-08 18:01:59 浏览: 57
在五子棋游戏中,评估函数通常是用来估计当前局面对玩家的优势程度,常用的方法有简单计数、启发式算法等。这里是一个简单的例子,展示如何使用常量加权评分法(也称“目”数)作为评估函数:
```cpp
#include <vector>
// 定义一些基本的得分值
const int EMPTY = 0; // 空位
const int BLACK_STONE = -1; // 黑子
const int WHITE_STONE = 1; // 白子
// 评估函数,每组连续同色棋子给予相应的分数
int evaluatePosition(const std::vector<std::vector<int>>& board) {
int score = 0;
for (size_t i = 0; i < board.size(); ++i) {
for (size_t j = 0; j < board[i].size(); ++j) {
if (board[i][j] == BLACK_STONE)
score -= countConsecutiveBlack(i, j, board);
else if (board[i][j] == WHITE_STONE)
score += countConsecutiveWhite(i, j, board);
}
}
return score;
}
// 计算某位置连续同色棋子的数量
int countConsecutive(int row, int col, const std::vector<std::vector<int>>& board) {
int count = 1;
inDirection(row, col, board, 0, 1); // 向右
inDirection(row, col, board, 0, -1); // 向左
inDirection(row, col, board, 1, 0); // 向下
inDirection(row, col, board, -1, 0); // 向上
return count;
}
void inDirection(int startRow, int startCol, const std::vector<std::vector<int>>& board, int dx, int dy) {
int n = countConsecutive(startRow, startCol, board);
while (startRow + dx >= 0 && startRow + dx < board.size() &&
startCol + dy >= 0 && startCol + dy < board[i].size() &&
board[startRow + dx][startCol + dy] == board[startRow][startCol]) {
++n;
++startRow + dx;
++startCol + dy;
}
}
// 示例用法
std::vector<std::vector<int>> board = {{0, 0, 0}, {0, 0, BLACK_STONE}, {WHITE_STONE, WHITE_STONE, 0}};
int result = evaluatePosition(board);
```
这个例子中,`evaluatePosition`函数遍历棋盘,根据每个位置的棋子颜色,计算其连续同色棋子的数量,并累加到总分。`countConsecutive`和`inDirection`用于递归地查找方向上的连续棋子。
阅读全文
相关推荐
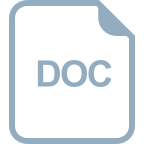
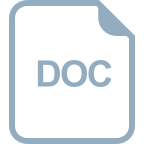
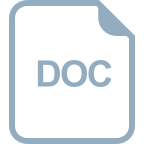

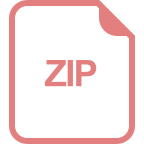
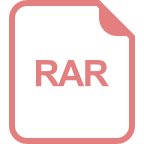
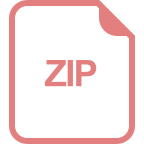
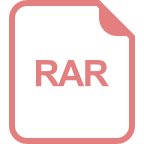
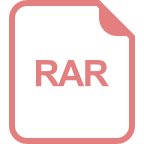
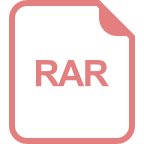
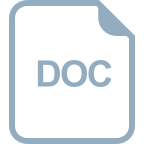
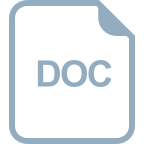
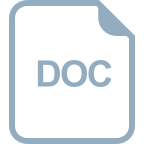
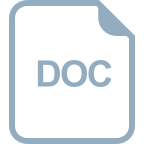
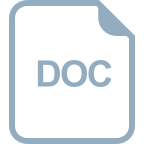
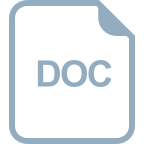
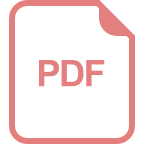
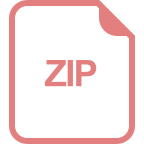
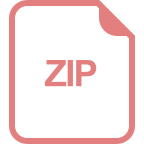