c#的list使用说明
时间: 2023-11-20 20:07:48 浏览: 40
C#中的List是一种动态数组,可以根据需要自动扩展和收缩。以下是使用List的一些基本操作:
1. 创建List对象:
List<T> list = new List<T>();
2. 添加元素:
list.Add(item);
3. 获取元素:
T item = list[index];
4. 修改元素:
list[index] = newItem;
5. 删除元素:
list.Remove(item);
list.RemoveAt(index);
6. 判断元素是否存在:
bool exists = list.Contains(item);
7. 获取元素个数:
int count = list.Count;
8. 清空List:
list.Clear();
相关问题
C# 检测 string 是否包含 list元素
在 C# 中,你可以使用 LINQ(Language Integrated Query)来检测一个字符串是否包含列表中的任何一个元素。下面是一个示例代码:
```csharp
using System;
using System.Linq;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> list = new List<string> { "apple", "banana", "orange" };
string input = "I like apples";
bool containsElement = list.Any(input.Contains);
Console.WriteLine(containsElement);
}
}
```
在上面的代码中,我们首先创建了一个包含若干字符串的列表 `list`,然后定义了一个待检测的字符串 `input`。通过使用 LINQ 的 `Any` 方法和 `input.Contains` 来检测是否有任何一个列表元素存在于输入字符串中。最后,我们输出结果。
请注意,上述代码仅检测了整个字符串是否包含列表中的元素,而不是仅仅匹配单词。如果你需要进行更复杂的匹配操作,请提供更详细的需求说明。
C#判断list所有存储对象是否相等,详细举例说明
假设我们有一个存储`Person`对象的List,需要判断其中所有对象是否相等。`Person`对象具有`Name`和`Age`两个属性。
```csharp
class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
```
我们可以使用上述两种方法进行判断。
方法一:使用LINQ中的`All()`方法
```csharp
List<Person> people = new List<Person>
{
new Person { Name = "Alice", Age = 20 },
new Person { Name = "Bob", Age = 25 },
new Person { Name = "Charlie", Age = 30 }
};
bool allEqual = people.All(x => x.Name == people[0].Name && x.Age == people[0].Age);
Console.WriteLine(allEqual); // false
```
方法二:重载`Equals()`方法
```csharp
class Person
{
public string Name { get; set; }
public int Age { get; set; }
public override bool Equals(object obj)
{
if (obj == null || GetType() != obj.GetType())
{
return false;
}
Person other = (Person)obj;
return (Name == other.Name) && (Age == other.Age);
}
}
List<Person> people = new List<Person>
{
new Person { Name = "Alice", Age = 20 },
new Person { Name = "Bob", Age = 25 },
new Person { Name = "Charlie", Age = 30 }
};
bool allEqual = people.All(x => x.Equals(people[0]));
Console.WriteLine(allEqual); // false
```
注意,在重载`Equals()`方法时,还需要重载`GetHashCode()`方法,以确保在使用哈希表等数据结构时,对象的相等性能够被正确判断。
相关推荐
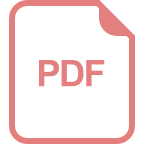
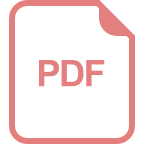












