ExcelUtil<MaterialsPropertyRefDto> util = new ExcelUtil<>(MaterialsPropertyRefDto.class); // param1:导出数据集合 param2:工作表名称 创建工作簿对象 HSSFWorkbook workbook = util.exportExcelExt(null, "material", fields); workbook.createName(); workbook.write(baos);
时间: 2024-04-16 08:25:54 浏览: 19
根据您提供的代码片段,我可以看出您在使用Excel框架进行导出操作。这段代码使用了一个名为`ExcelUtil`的工具类来导出Excel文件,并将数据写入到`HSSFWorkbook`对象中。
根据代码中的注释,`param1`表示导出的数据集合,`param2`表示工作表的名称,`fields`可能是用于指定导出字段的参数。
接下来,代码调用了`util.exportExcelExt()`方法来执行导出操作,返回一个`HSSFWorkbook`对象。然后,通过调用`createName()`方法创建了一个名称,并使用`workbook.write(baos)`将数据写入到一个输出流(例如`ByteArrayOutputStream`)中。
根据您的需求,您可能需要提供数据集合和工作表名称作为参数来导出实际的数据。如果您需要更具体的帮助,请提供更多代码或详细描述您遇到的问题,我将尽力协助您解决。
相关问题
Map<String, List<PortReportResultVo>> relayLinkAndPortMap = devPortReportByEs.getRelayLinkAndPortMap(); 遍历这个map,key为表头,value为数据,在一个sheet页中用easyExcel实现,导包也展示
好的,你可以参考以下代码实现:
```
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.annotation.ExcelProperty;
import com.alibaba.excel.metadata.BaseRowModel;
import com.alibaba.excel.metadata.Sheet;
import com.alibaba.excel.support.ExcelTypeEnum;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public class ExcelUtil {
/**
* 导出到Excel
*
* @param outputStream 输出流
* @param dataMap 数据集合,key为表头,value为数据
* @throws IOException
*/
public static void exportExcel(OutputStream outputStream, Map<String, List<PortReportResultVo>> dataMap) throws IOException {
List<PortReportResultVo> dataList = new ArrayList<>();
for (Map.Entry<String, List<PortReportResultVo>> entry : dataMap.entrySet()) {
// 添加表头
PortReportResultVo header = new PortReportResultVo();
header.setPortCode(entry.getKey());
dataList.add(header);
// 添加数据
List<PortReportResultVo> list = entry.getValue();
dataList.addAll(list);
}
// 导出Excel
EasyExcel.write(outputStream, PortReportResultVo.class)
.excelType(ExcelTypeEnum.XLSX)
.sheet("Sheet1")
.doWrite(dataList);
}
/**
* 导出到文件
*
* @param filePath 文件路径
* @param dataMap 数据集合,key为表头,value为数据
* @throws IOException
*/
public static void exportExcel(String filePath, Map<String, List<PortReportResultVo>> dataMap) throws IOException {
try (OutputStream outputStream = new FileOutputStream(filePath)) {
exportExcel(outputStream, dataMap);
}
}
/**
* 导出到文件
*
* @param filePath 文件路径
* @param dataMap 数据集合,key为表头,value为数据
* @throws IOException
*/
public static void exportExcel(String filePath, Map<String, List<PortReportResultVo>> dataMap, String sheetName) throws IOException {
try (OutputStream outputStream = new FileOutputStream(filePath)) {
exportExcel(outputStream, dataMap, sheetName);
}
}
/**
* 导出到Excel
*
* @param outputStream 输出流
* @param dataMap 数据集合,key为表头,value为数据
* @param sheetName sheet页名称
* @throws IOException
*/
public static void exportExcel(OutputStream outputStream, Map<String, List<PortReportResultVo>> dataMap, String sheetName) throws IOException {
List<PortReportResultVo> dataList = new ArrayList<>();
for (Map.Entry<String, List<PortReportResultVo>> entry : dataMap.entrySet()) {
// 添加表头
PortReportResultVo header = new PortReportResultVo();
header.setPortCode(entry.getKey());
dataList.add(header);
// 添加数据
List<PortReportResultVo> list = entry.getValue();
dataList.addAll(list);
}
// 导出Excel
Sheet sheet = new Sheet(1, 0, PortReportResultVo.class);
sheet.setSheetName(sheetName);
EasyExcel.write(outputStream, PortReportResultVo.class)
.excelType(ExcelTypeEnum.XLSX)
.sheet(sheet)
.doWrite(dataList);
}
}
/**
* Excel行数据类
*/
class PortReportResultVo extends BaseRowModel {
@ExcelProperty(value = "端口编码", index = 0)
private String portCode;
@ExcelProperty(value = "端口名称", index = 1)
private String portName;
@ExcelProperty(value = "设备编码", index = 2)
private String deviceCode;
@ExcelProperty(value = "设备名称", index = 3)
private String deviceName;
// 省略getter/setter方法
}
```
其中,PortReportResultVo类为Excel行数据类,用于存储一行数据。ExcelUtil类中的exportExcel方法可以将Map<String, List<PortReportResultVo>>数据导出为一个Excel文件。你可以根据需要修改ExcelUtil类中的代码,并添加相应的依赖包,比如:
```
<dependencies>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>2.2.4</version>
</dependency>
</dependencies>
```
java实现以下功能:扫描指定路径下的excel文件,若无则进行提示,若存在excel文件则循环读取所有的文件,利用实体类@Excel注解的方式读取excel的数据,将读取到的数据插入oracle数据库指定的表中
首先需要引入poi、poi-ooxml、commons-collections4、commons-lang3、commons-beanutils、oracle-jdbc驱动等相关依赖。
然后,可以编写一个ExcelUtil工具类,用于读取Excel文件并转换成对象列表:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.text.DecimalFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.apache.commons.beanutils.BeanUtils;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.poi.hssf.usermodel.HSSFDateUtil;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.ss.usermodel.DateUtil;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelUtil {
/**
* 读取Excel文件并转换成对象列表
*
* @param file Excel文件
* @param clazz 对象类型
* @return 对象列表
*/
public static <T> List<T> readExcel(File file, Class<T> clazz) throws IOException {
List<T> list = new ArrayList<>();
try (InputStream is = new FileInputStream(file); Workbook workbook = new XSSFWorkbook(is)) {
Sheet sheet = workbook.getSheetAt(0);
if (sheet == null) {
return list;
}
// 获取表头行
Row headerRow = sheet.getRow(0);
if (headerRow == null) {
return list;
}
// 获取表头字段名
List<String> headerFieldNames = new ArrayList<>();
for (int i = 0; i < headerRow.getLastCellNum(); i++) {
Cell cell = headerRow.getCell(i);
if (cell == null || cell.getCellType() == CellType.BLANK) {
headerFieldNames.add(null);
} else {
headerFieldNames.add(cell.getStringCellValue().trim());
}
}
// 获取字段映射关系
List<FieldMapping> fieldMappings = getFieldMappings(clazz, headerFieldNames);
// 遍历每一行数据
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
if (row == null) {
continue;
}
T obj = clazz.newInstance();
// 遍历每一个单元格数据
for (FieldMapping fieldMapping : fieldMappings) {
Cell cell = row.getCell(fieldMapping.getIndex());
if (cell == null || cell.getCellType() == CellType.BLANK) {
continue;
}
setFieldValue(obj, fieldMapping.getField(), cell);
}
list.add(obj);
}
} catch (Exception e) {
throw new RuntimeException("Read Excel error", e);
}
return list;
}
/**
* 获取字段映射关系
*
* @param clazz 对象类型
* @param headerFieldNames 表头字段名列表
* @return 字段映射关系列表
*/
private static List<FieldMapping> getFieldMappings(Class<?> clazz, List<String> headerFieldNames) {
List<FieldMapping> fieldMappings = new ArrayList<>();
for (Field field : clazz.getDeclaredFields()) {
Excel excel = field.getAnnotation(Excel.class);
if (excel == null) {
continue;
}
String fieldName = StringUtils.isBlank(excel.fieldName()) ? field.getName() : excel.fieldName();
int index = headerFieldNames.indexOf(fieldName);
if (index < 0) {
throw new RuntimeException("Excel header does not contain field: " + fieldName);
}
fieldMappings.add(new FieldMapping(field, index));
}
return fieldMappings;
}
/**
* 设置对象字段值
*/
private static void setFieldValue(Object obj, Field field, Cell cell) throws Exception {
String cellValue = getCellValue(cell);
Class<?> fieldType = field.getType();
if (StringUtils.isBlank(cellValue)) {
BeanUtils.setProperty(obj, field.getName(), null);
return;
}
if (fieldType == String.class) {
BeanUtils.setProperty(obj, field.getName(), cellValue);
} else if (fieldType == Integer.TYPE || fieldType == Integer.class) {
BeanUtils.setProperty(obj, field.getName(), Integer.parseInt(cellValue));
} else if (fieldType == Long.TYPE || fieldType == Long.class) {
BeanUtils.setProperty(obj, field.getName(), Long.parseLong(cellValue));
} else if (fieldType == Float.TYPE || fieldType == Float.class) {
BeanUtils.setProperty(obj, field.getName(), Float.parseFloat(cellValue));
} else if (fieldType == Double.TYPE || fieldType == Double.class) {
BeanUtils.setProperty(obj, field.getName(), Double.parseDouble(cellValue));
} else if (fieldType == Date.class) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
BeanUtils.setProperty(obj, field.getName(), sdf.parse(cellValue));
} else {
throw new RuntimeException("Unsupported field type: " + fieldType.getName());
}
}
/**
* 获取单元格值
*/
private static String getCellValue(Cell cell) {
if (cell == null) {
return null;
}
DecimalFormat df = new DecimalFormat("#");
switch (cell.getCellType()) {
case STRING:
return cell.getStringCellValue().trim();
case NUMERIC:
if (DateUtil.isCellDateFormatted(cell)) {
Date date = HSSFDateUtil.getJavaDate(cell.getNumericCellValue());
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
return sdf.format(date);
} else {
return df.format(cell.getNumericCellValue());
}
case BOOLEAN:
return String.valueOf(cell.getBooleanCellValue());
case FORMULA:
return getCellValue(cell.getCachedFormulaResultType() == CellType.NUMERIC ? cell : cell.getSheet().getRow(cell.getRowIndex() + 1).getCell(cell.getColumnIndex()));
default:
return null;
}
}
/**
* 字段映射关系
*/
private static class FieldMapping {
private Field field;
private int index;
public FieldMapping(Field field, int index) {
this.field = field;
this.index = index;
}
public Field getField() {
return field;
}
public int getIndex() {
return index;
}
}
}
```
接着,可以定义一个Excel注解用于标记实体类的属性,以及一个扫描指定路径下Excel文件并插入数据库的方法:
```java
import java.io.File;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.util.List;
public class ExcelDemo {
@Excel(fieldName = "姓名")
private String name;
@Excel(fieldName = "年龄")
private int age;
@Excel(fieldName = "性别")
private String gender;
@Excel(fieldName = "职业")
private String occupation;
public static void main(String[] args) throws IOException {
String excelDir = "path/to/excel/dir";
String jdbcUrl = "jdbc:oracle:thin:@localhost:1521:orcl";
String username = "username";
String password = "password";
File dir = new File(excelDir);
if (!dir.exists() || !dir.isDirectory()) {
System.out.println("Excel directory does not exist");
return;
}
File[] files = dir.listFiles((dir1, name) -> name.toLowerCase().endsWith(".xlsx"));
if (files == null || files.length == 0) {
System.out.println("No Excel file found in directory");
return;
}
try (Connection conn = DriverManager.getConnection(jdbcUrl, username, password)) {
for (File file : files) {
List<ExcelDemo> dataList = ExcelUtil.readExcel(file, ExcelDemo.class);
if (CollectionUtils.isEmpty(dataList)) {
continue;
}
String sql = "INSERT INTO T_EXCEL_DEMO (NAME, AGE, GENDER, OCCUPATION) VALUES (?, ?, ?, ?)";
try (PreparedStatement pstmt = conn.prepareStatement(sql)) {
for (ExcelDemo data : dataList) {
pstmt.setString(1, data.name);
pstmt.setInt(2, data.age);
pstmt.setString(3, data.gender);
pstmt.setString(4, data.occupation);
pstmt.executeUpdate();
}
}
}
} catch (Exception e) {
throw new RuntimeException("Insert data error", e);
}
}
}
```
其中,@Excel注解可以用于标记实体类的属性,指定其对应的Excel表头字段名:
```java
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface Excel {
String fieldName();
}
```
相关推荐
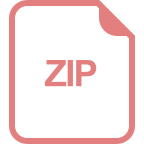









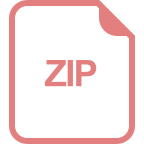