opencv读取文件夹图片,并将图片的地址保存在一个string[]数组里
时间: 2024-09-06 14:01:53 浏览: 61
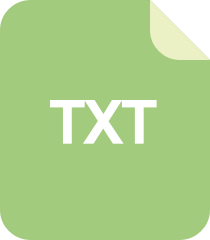
opencv 读取文件夹中的多幅图片并保存

在OpenCV库中,你可以使用`cv::glob`函数来获取指定文件夹下的所有图片路径,然后把这些路径存入`std::vector<std::string>`或者`string[]`数组。以下是一个简单的步骤示例:
```cpp
#include <opencv2/opencv.hpp>
#include <filesystem>
std::vector<std::string> imagesPaths;
// 定义文件夹路径
std::string folderPath = "path_to_your_folder";
// 使用cv::glob获取文件夹内所有.jpg或.png类型的图片路径
std::vector<cv::String> filePaths = cv::glob(folderPath + "/*.jpg"); // 或者 "*.png"
// 将路径添加到字符串向量中
for (const auto& filePath : filePaths) {
imagesPaths.push_back(filePath);
}
// 如果需要转换为string数组,可以这样做:
std::string* strArray = new std::string[imagesPaths.size()];
for (size_t i = 0; i < imagesPaths.size(); i++) {
strArray[i] = imagesPaths[i];
}
阅读全文
相关推荐
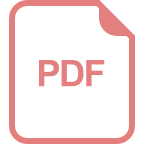

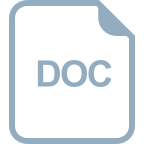
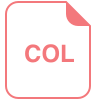
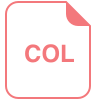
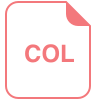
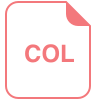
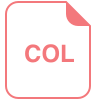
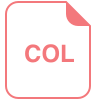


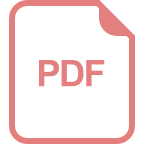
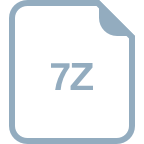
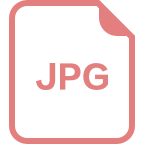