单链表的基本操作实现
时间: 2024-10-13 13:01:54 浏览: 24
单链表是一种线性的数据结构,它由一系列节点组成,每个节点包含两个部分:存储数据的变量和指向下一个节点的引用。单链表的基本操作通常包括以下几个:
1. **创建和初始化**:创建一个新节点,并将其头指针设置为null表示空链表。
```java
Node createNode(int data) {
Node newNode = new Node(data);
newNode.next = null;
return newNode;
}
```
2. **添加元素**:在链表的末尾添加一个新节点。如果链表为空,则直接将头指针指向新节点。
```java
void append(Node head, int data) {
if (head == null) {
head = createNode(data);
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = createNode(data);
}
}
```
3. **删除元素**:根据给定的数据值,从链表中删除第一个找到该值的节点。如果链表为空或需要删除的节点是头节点,特殊处理。
```java
void deleteNode(Node head, int key) {
if (head == null || head.data == key) {
// 处理头节点
head = head.next;
} else {
Node current = head;
while (current.next != null && current.next.data != key) {
current = current.next;
}
if (current.next != null) {
current.next = current.next.next;
}
}
}
```
4. **查找元素**:遍历链表,查找指定的数据值。
```java
Node search(Node head, int key) {
Node current = head;
while (current != null) {
if (current.data == key) {
return current;
}
current = current.next;
}
return null; // 没找到
}
```
5. **打印链表**:顺序访问链表并输出所有节点的数据。
```java
void printList(Node head) {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
```
阅读全文
相关推荐
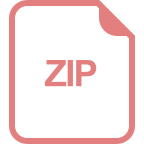
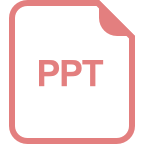
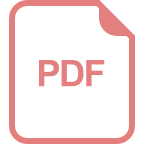
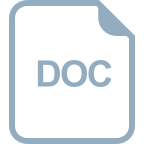
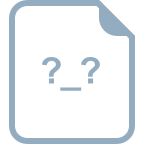
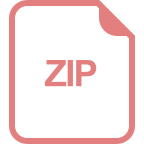
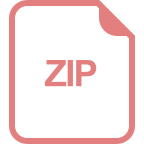
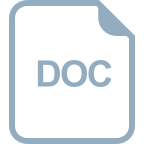
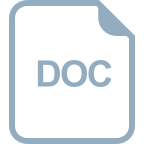
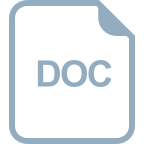

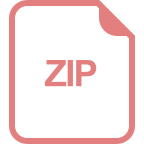
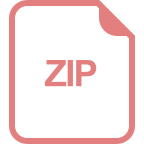
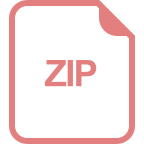
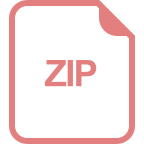