c++map遍历用途
时间: 2024-10-22 11:15:24 浏览: 40
C++中的`std::map`是一个关联容器,它按照键值对的方式存储数据,其中每个键都是唯一的。遍历`map`的主要用途有:
1. **访问元素**:你可以通过键来查找对应的值,遍历过程可以让你逐个获取并处理存储的数据。
2. **数据检查**:检查map中是否存在某个键,或者统计元素数量。
3. **修改元素**:如果需要更新已有键对应的数据,遍历过程中可以直接找到并修改。
4. **初始化和清理**:在创建新map时,遍历旧数据结构并插入新的键值对是一种常用方法。同样,当不再需要某些数据时,也可以删除对应的键值对。
5. **算法基础**:作为许多高级算法的基础,如搜索、排序等,遍历map常常用于实现特定功能。
常见的遍历方法包括使用迭代器(`map::begin()` 和 `map::end()`)或者直接调用成员函数`map::find()`、`map::begin()` 和 `map::upper_bound()`。以下是使用迭代器的基本示例:
```cpp
for (auto it = map.begin(); it != map.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
```
相关问题
c++ map 遍历
你可以使用迭代器来遍历 C++ 的 map。下面是一个示例代码:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "apple";
myMap[2] = "banana";
myMap[3] = "orange";
// 使用迭代器进行遍历
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
这段代码创建了一个 `map`,并添加了几个键值对。然后使用迭代器 `it` 遍历 `myMap`,输出每个键值对的信息。输出结果为:
```
Key: 1, Value: apple
Key: 2, Value: banana
Key: 3, Value: orange
```
C++ map遍历
在C++中,我们可以使用迭代器来遍历map。下面是一个示例代码:
```c++
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap = {{"apple", 5}, {"banana", 3}, {"orange", 2}};
// 使用 auto 关键字来声明迭代器变量
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << " : " << it->second << std::endl;
}
return 0;
}
```
输出结果为:
```
apple : 5
banana : 3
orange : 2
```
在循环中,我们使用auto关键字来声明迭代器变量it,其类型为std::map<std::string, int>::iterator。循环条件为it != myMap.end(),即迭代器未到达map的末尾。在每次循环中,我们使用it->first和it->second来访问map中的键和值,然后输出它们。最后,我们通过++it来将迭代器移动到下一个元素。
阅读全文
相关推荐
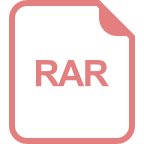
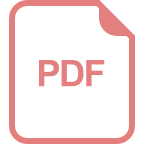
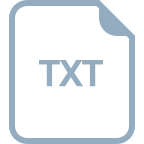













