c++11 map 遍历
时间: 2023-11-14 09:11:01 浏览: 114
在C++11中,有三种方法可以遍历map:
1. 迭代器(iterator):使用auto关键字定义迭代器,通过循环遍历map中的键值对,可以通过it->first和it->second分别获取键和值。
2. 范围for语句(range for):使用auto关键字定义一个引用,通过循环遍历map中的值,可以直接获取值。
3. 结构化绑定声明和范围for语句(structured binding declaration && range for):使用auto关键字定义一个引用,通过循环遍历map中的键值对,可以通过[key, value]获取键和值。
如果只需要遍历map中的键或值,可以使用占位符_1来代替不需要的变量。
相关问题
c++ 嵌套map遍历
以下是C++中嵌套map的遍历方法:
```cpp
#include <iostream>
#include <map>
using namespace std;
int main() {
map<int, map<int, string>> nestedMap;
nestedMap[0][0] = "hello";
nestedMap[0][1] = "world";
nestedMap[1][0] = "foo";
nestedMap[1][1] = "bar";
for (auto& outerPair : nestedMap) {
int outerKey = outerPair.first;
map<int, string>& innerMap = outerPair.second; for (auto& innerPair : innerMap) {
int innerKey = innerPair.first;
string value = innerPair.second;
cout << "outer key: " << outerKey << ", inner key: " << innerKey << ", value: " << value << endl;
}
}
return 0;
}
```
输出结果为:
```
outer key: 0, inner key: 0, value: hello
outer key: 0, inner key: 1, value: world
outer key: 1, inner key: 0, value: foo
outer key: 1, inner key: 1, value: bar
```
c++ unordered map遍历
在C++中,你可以使用范围循环(range-based for loop)来遍历`std::unordered_map`(无序映射)。下面是一个示例代码,展示了如何遍历一个`std::unordered_map`并打印出其中的键和值:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap = {
{1, "apple"},
{2, "banana"},
{3, "orange"}
};
// 使用范围循环遍历unordered_map
for (const auto& pair : myMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
return 0;
}
```
在这个示例中,我们创建了一个`std::unordered_map`,其中键是整数,值是字符串。然后,我们使用范围循环来遍历这个无序映射。在每次循环中,`pair`被绑定到当前键值对,并可以通过`pair.first`和`pair.second`访问键和值。我们将它们打印出来。
运行这段代码,你会得到如下输出:
```
Key: 1, Value: apple
Key: 2, Value: banana
Key: 3, Value: orange
```
这样就完成了对`std::unordered_map`的遍历。范围循环使得代码更加简洁和易读。希望这个示例对你有帮助!如果你还有其他问题,请随时提问。
相关推荐
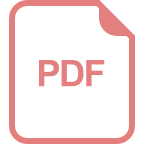
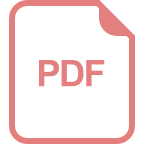
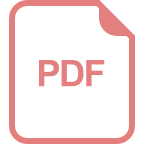
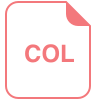









