C++ map遍历
时间: 2023-06-28 14:07:15 浏览: 50
在C++中,我们可以使用迭代器来遍历map。下面是一个示例代码:
```c++
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap = {{"apple", 5}, {"banana", 3}, {"orange", 2}};
// 使用 auto 关键字来声明迭代器变量
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << " : " << it->second << std::endl;
}
return 0;
}
```
输出结果为:
```
apple : 5
banana : 3
orange : 2
```
在循环中,我们使用auto关键字来声明迭代器变量it,其类型为std::map<std::string, int>::iterator。循环条件为it != myMap.end(),即迭代器未到达map的末尾。在每次循环中,我们使用it->first和it->second来访问map中的键和值,然后输出它们。最后,我们通过++it来将迭代器移动到下一个元素。
相关问题
c++ map 遍历
在C++中,可以使用迭代器、range for循环和C++17的结构化绑定来遍历map。
一种遍历方式是使用迭代器。可以通过使用map的begin()和end()函数来获取map的起始和结束迭代器,然后使用一个循环来迭代访问每个元素。例如,可以使用以下代码进行遍历:
#include <map>
#include <iostream>
int main() {
std::map<int, std::string> mapStudent;
mapStudent = "student_one";
mapStudent = "student_two";
mapStudent = "student_three";
std::map<int, std::string>::iterator iter; // 声明迭代器
for (iter = mapStudent.begin(); iter != mapStudent.end(); iter++) {
std::cout << iter->first << " " << iter->second << std::endl;
}
return 0;
}
另一种遍历方式是使用C++11及以上版本的range for循环。可以直接使用auto关键字来声明一个变量来表示每个键值对,并通过range for循环遍历map。以下是示例代码:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> mp;
mp["张三"] = 20;
mp["李四"] = 18;
mp["王五"] = 30;
std::cout << "方式一、迭代器" << std::endl;
for (auto it = mp.begin(); it != mp.end(); it++) {
std::cout << it->first << " " << it->second << std::endl;
}
std::cout << "\n方法二、range for" << std::endl;
for (auto it : mp) {
std::cout << it.first << " " << it.second << std::endl;
}
std::cout << "\n方法三" << std::endl;
for (auto [key, val] : mp) {
std::cout << key << " " << val << std::endl;
}
return 0;
}
另外还可以使用简单的for循环和size()函数来遍历map。以下是示例代码:
#include <map>
#include <iostream>
int main() {
std::map<int, std::string> mapStudent;
mapStudent = "student_one";
mapStudent = "student_two";
mapStudent = "student_three";
int iSize = mapStudent.size();
for (int i = 1; i <= iSize; i++) {
std::cout << i << " " << mapStudent[i] << std::endl;
}
return 0;
}
以上是C++中遍历map的几种方式。可以根据实际情况选择合适的方式来遍历map。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [C++中map的遍历](https://blog.csdn.net/chengqiuming/article/details/89815814)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* [C++ map遍历的几种方式](https://blog.csdn.net/VariatioZbw/article/details/124947520)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
c++map遍历auto
C++中的std::map是一个关联容器,它提供了一种将键值对映射起来的方式。如果你想要遍历一个std::map并使用auto关键字来推断迭代器的类型,你可以使用范围遍历循环(range-based for loop)进行遍历操作。下面是一个示例代码:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "apple";
myMap[2] = "banana";
myMap[3] = "orange";
for (const auto& pair : myMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
return 0;
}
```
在这个例子中,我们创建了一个std::map对象myMap,并给它添加了三对键值对。然后,我们使用范围遍历循环来遍历整个map。循环中的每个元素都被绑定到名为pair的变量中,其中pair.first表示键,pair.second表示值。我们使用auto关键字来推断迭代器的类型,并使用const修饰符来确保不修改元素。
输出结果为:
```
Key: 1, Value: apple
Key: 2, Value: banana
Key: 3, Value: orange
```
这样就完成了对std::map的遍历操作。希望能帮到你!如果还有其他问题,请继续提问。
相关推荐
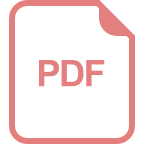












