什么是拓扑排序?如何实现拓扑排序?
时间: 2023-11-20 18:57:52 浏览: 105
拓扑排序是一种对有向无环图进行排序的算法,它将图中的节点按照它们之间的依赖关系进行排序,即若存在一条从节点A到节点B的有向边,则在排序结果中节点A出现在节点B之前。拓扑排序可以用于判断有向图中是否存在环,若存在环则无法进行拓扑排序。
实现拓扑排序的一种常见方法是使用Kahn算法,具体步骤如下:
1. 统计每个节点的入度(即有多少条有向边指向该节点),并将入度为0的节点加入一个队列中。
2. 从队列中取出一个节点,将其加入拓扑序列中,并将该节点指向的所有节点的入度减1。
3. 若某个节点的入度减为0,则将其加入队列中。
4. 重复步骤2和3,直到队列为空。
下面是一个Python实现的例子:
```python
from collections import deque
def topological_sort(graph):
# 统计每个节点的入度
in_degree = {node: 0 for node in graph}
for node in graph:
for neighbor in graph[node]:
in_degree[neighbor] += 1
# 将入度为0的节点加入队列
queue = deque([node for node in graph if in_degree[node] == 0])
# 依次取出队列中的节点,并将其指向的节点的入度减1
result = []
while queue:
node = queue.popleft()
result.append(node)
for neighbor in graph[node]:
in_degree[neighbor] -= 1
if in_degree[neighbor] == 0:
queue.append(neighbor)
# 若存在环,则无法进行拓扑排序
if len(result) != len(graph):
return None
else:
return result
```
阅读全文
相关推荐


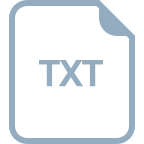
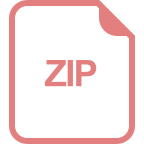
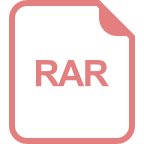
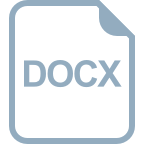
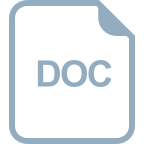
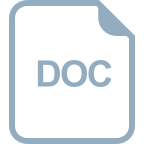
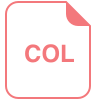




