编写一个C程序,输入一个年份,判断这个年份是否是闰年。(能被4整除但不能被100 整除,或者能被400整除的年份
时间: 2024-10-20 21:08:30 浏览: 42
你可以使用C语言编写如下的程序来判断一个年份是否是闰年。这里是一个简单的函数实现:
```c
#include <stdio.h>
// 判断闰年的函数
int is_leap_year(int year) {
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0)
return 1; // 能被400整除的是闰年
else
return 0; // 否则不是闰年
}
else
return 1; // 能被4整除但不能被100整除的是闰年
}
else
return 0; // 不满足条件,所以不是闰年
}
int main() {
int year;
printf("请输入一个年份:");
scanf("%d", &year);
if (is_leap_year(year))
printf("%d年是闰年。\n", year);
else
printf("%d年不是闰年。\n", year);
return 0;
}
```
在这个程序中,`is_leap_year`函数接收一个年份作为参数,并检查其是否满足闰年的规则。主函数中从用户获取输入,然后调用该函数并输出结果。
相关问题
题目三:判断闰年 要求:编写一个C语言程序,输入一个年份,判断该年份是否为闰年。闰年的判断条件是:能被4整除但不能被100整除,或者能被400整除。
题目三:在C语言中,判断闰年的逻辑可以通过以下步骤实现:
```c
#include <stdio.h>
int is_leap_year(int year) {
// 判断闰年的条件
if (year % 4 == 0) {
if (year % 100 != 0 || year % 400 == 0) {
return 1; // 是闰年
}
} else {
return 0; // 不是闰年
}
}
int main() {
int year;
printf("请输入一个年份:");
scanf("%d", &year);
if (is_leap_year(year)) {
printf("%d年是闰年。\n", year);
} else {
printf("%d年不是闰年。\n", year);
}
return 0;
}
```
在这个程序中,`is_leap_year`函数接收一个年份作为参数,通过一系列的if...else条件判断来确定它是否满足闰年的规则。主函数则读取用户输入的年份并调用这个函数。
已知能被 4 整除但不能被 100 整除或者能被 400 整除的年份是闰年。请编一个c程序要求输入一个年份,判断这个年份是否是闰年
下面是一个用C语言编写的程序,根据输入的年份判断是否为闰年:
```c
#include <stdio.h>
int main() {
int year;
printf("请输入一个年份:");
scanf("%d", &year);
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
printf("%d年是闰年\n", year);
} else {
printf("%d年不是闰年\n", year);
}
return 0;
}
```
你可以将上述代码保存为一个以`.c`为后缀的文件,然后使用C编译器进行编译和运行。程序会要求用户输入一个年份,然后根据闰年的判断条件判断并输出是否为闰年。如果是闰年,输出"年份是闰年",否则输出"年份不是闰年"。
阅读全文
相关推荐
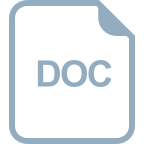
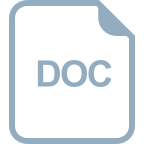
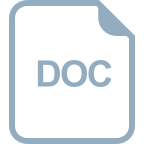













