c语言编程实现建立线程多个线程,主线程休眠。该程序使用函数什么启动多个线程?函数ThreadFun执行的流程?主函数执行的流程?
时间: 2024-12-12 22:22:56 浏览: 7
在C语言中,创建并管理线程通常需要用到`pthread.h`库。为了实现主线程休眠并启动多个子线程,可以按照以下步骤操作:
1. **包含头文件**:
首先,在程序开始处包含`pthread.h`和其他必要的头文件。
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h> // 包含sleep函数
```
2. **结构体和函数声明**:
定义一个用于线程数据的结构体,并声明处理线程任务的函数`ThreadFun`。
```c
typedef void* (*ThreadFunc)(void*);
struct thread_data {
int id; // 线程ID
};
// 定义线程执行的函数
ThreadFunc ThreadFun(void *arg);
```
3. **创建线程**:
使用`pthread_create()`函数创建新线程,它接受四个参数:返回值、线程标识符、线程函数以及线程数据。
```c
int create_thread(pthread_t *thread_id, ThreadFunc func, void *data) {
if (pthread_create(thread_id, NULL, func, data)) { // 创建新线程
perror("Error creating thread");
exit(EXIT_FAILURE);
}
return 0;
}
// 主线程创建子线程
void main_func() {
pthread_t threads[ NUM_THREADS ]; // 存储线程ID的数组
struct thread_data thread_args[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; ++i) {
thread_args[i].id = i;
if (create_thread(&threads[i], ThreadFun, &thread_args[i])) {
// 处理错误
}
}
}
```
4. **线程函数`ThreadFun`的执行流程**:
- 函数接收`void *arg`作为参数,实际上是一个指向`thread_data`结构体的指针。
- 在函数内部,通过解引用获取到具体的`thread_data`结构体。
- 执行特定的任务(如循环、计算等),然后结束线程。
5. **主线程的执行流程**:
- 主线程创建所有子线程后,继续执行接下来的操作。
- 可能会有一个睡眠调用`sleep(SECONDS_TO_SLEEP)`来让主线程暂停一段时间,让子线程有机会运行。
- 最后,当所有需要的操作完成或者程序结束时,主线程可以遍历`threads`数组,调用`pthread_join()`等待每个子线程结束,然后再退出。
```c
void main_func() {
// ...创建子线程
// 主线程休眠
sleep(SECONDS_TO_SLEEP);
// 等待子线程结束
for (int i = 0; i < NUM_THREADS; ++i) {
pthread_join(threads[i], NULL);
}
printf("All threads finished.\n");
exit(0);
}
```
阅读全文
相关推荐
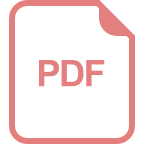
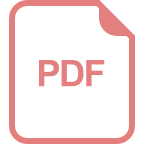
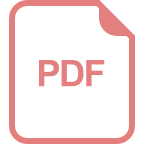
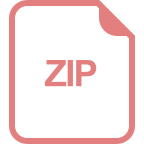
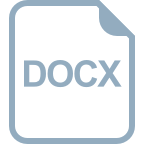
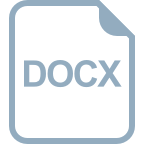
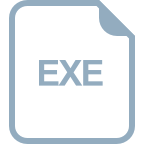
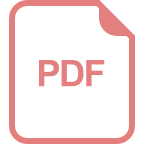
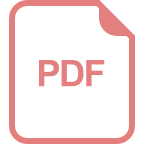
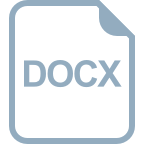
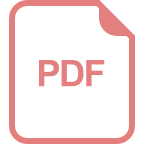
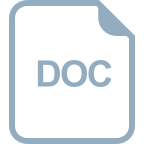
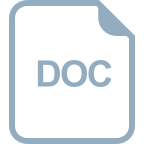
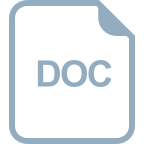
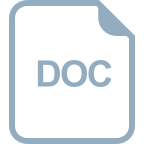


