带头结点的单链表插入操作C++
时间: 2024-11-04 18:16:16 浏览: 19
带头结点的单链表在C++中插入操作通常涉及以下几个步骤:
1. **定义结构体**:首先,定义一个包含数据域和指向下一个节点指针的链表节点结构体,例如:
```cpp
struct ListNode {
int data; // 数据域
ListNode* next; // 指向下一个节点的指针
};
```
2. **创建头结点**:如果链表为空,需要先创建一个空的头结点,`ListNode *head = new ListNode();`
3. **插入操作**:假设我们要在特定位置插入新节点,可以分为以下情况:
- 插入到开头:创建新节点并将`next`指针指向当前头结点,然后更新头结点为新节点,`head = new ListNode(data); head->next = head;`
- 插入到中间或结尾:遍历链表找到插入位置,对于每个节点,将`newNode.next`设置为当前节点的`next`,然后将当前节点的`next`指向新节点。
4. **迭代实现**:
```cpp
void insertNode(int data, ListNode*& head, int position) {
if (position == 0) { // 插入到头部
ListNode newNode = ListNode{data};
newNode.next = head;
head = &newNode;
} else {
ListNode* current = head;
for (int i = 0; i < position - 1 && current != nullptr; ++i)
current = current->next;
if (current != nullptr) {
ListNode newNode = ListNode{data};
newNode.next = current->next;
current->next = &newNode;
}
}
}
```
5. **结束操作**:别忘了处理特殊情况进行边界检查,如当`position < 0`或链表为空时。
阅读全文
相关推荐
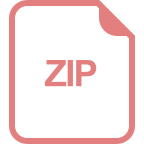
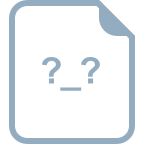
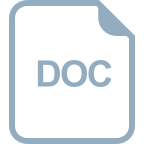









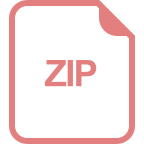





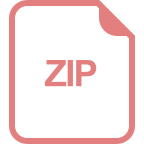