c语言图书馆管理系统源代码
时间: 2023-12-19 17:31:31 浏览: 80
以下是一个简单的C语言图书管理系统的源代码,包括添加图书、删除图书、查找图书和显示所有图书等功能。代码中使用了结构体和动态内存分配等C语言基础知识。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 50
#define MAX_AUTHOR_LEN 50
#define MAX_BOOKS 100
struct Book {
char name[MAX_NAME_LEN];
char author[MAX_AUTHOR_LEN];
int id;
};
struct Library {
struct Book* books[MAX_BOOKS];
int num_books;
};
void add_book(struct Library* lib, char* name, char* author, int id) {
if (lib->num_books >= MAX_BOOKS) {
printf("The library is full!\n");
return;
}
struct Book* book = (struct Book*)malloc(sizeof(struct Book));
strcpy(book->name, name);
strcpy(book->author, author);
book->id = id;
lib->books[lib->num_books] = book;
lib->num_books++;
printf("Book added successfully!\n");
}
void remove_book(struct Library* lib, int id) {
int i;
for (i = 0; i < lib->num_books; i++) {
if (lib->books[i]->id == id) {
free(lib->books[i]);
lib->books[i] = NULL;
printf("Book removed successfully!\n");
return;
}
}
printf("Book not found!\n");
}
void find_book(struct Library* lib, char* name) {
int i;
for (i = 0; i < lib->num_books; i++) {
if (strcmp(lib->books[i]->name, name) == 0) {
printf("Book found! Author: %s, ID: %d\n", lib->books[i]->author, lib->books[i]->id);
return;
}
}
printf("Book not found!\n");
}
void show_all_books(struct Library* lib) {
int i;
for (i = 0; i < lib->num_books; i++) {
printf("Name: %s, Author: %s, ID: %d\n", lib->books[i]->name, lib->books[i]->author, lib->books[i]->id);
}
}
int main() {
struct Library lib;
lib.num_books = 0;
char name[MAX_NAME_LEN];
char author[MAX_AUTHOR_LEN];
int id;
int choice;
while (1) {
printf("1. Add book\n");
printf("2. Remove book\n");
printf("3. Find book\n");
printf("4. Show all books\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter book name: ");
scanf("%s", name);
printf("Enter author name: ");
scanf("%s", author);
printf("Enter book ID: ");
scanf("%d", &id);
add_book(&lib, name, author, id);
break;
case 2:
printf("Enter book ID: ");
scanf("%d", &id);
remove_book(&lib, id);
break;
case 3:
printf("Enter book name: ");
scanf("%s", name);
find_book(&lib, name);
break;
case 4:
show_all_books(&lib);
break;
case 5:
exit(0);
default:
printf("Invalid choice!\n");
}
}
return 0;
}
```
阅读全文
相关推荐
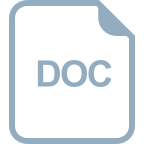
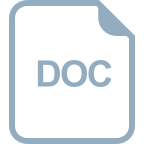
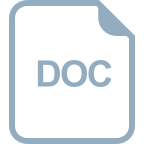
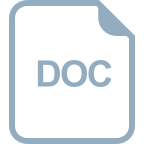
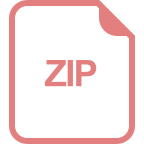
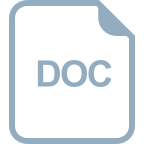

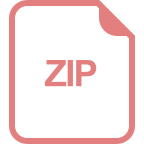
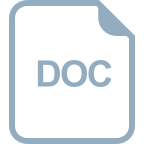
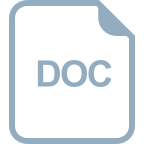
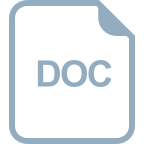
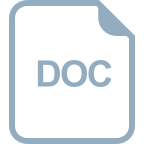
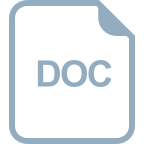
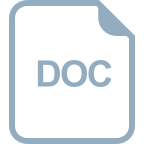
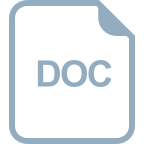

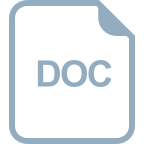