.编写程序、完成如下功能:从键盘输入任意年份vear,判别该年份是否闰年。 俞入:从键盘任意输入一个年份。 输出:根据是否闰年进行输出,是输出"****是闰年!",否输出"****不是闰年!”,其中****为当前输入的年份。
时间: 2024-10-07 20:07:39 浏览: 26
为了编写这个程序,你可以使用Python语言。这里是一个简单的函数实现,它会根据闰年的规则判断用户输入的年份是否为闰年:
```python
def is_leap_year(year):
if year % 4 != 0:
return False
elif year % 100 != 0:
return True
elif year % 400 != 0:
return False
else:
return True
year = int(input("请输入一个年份: "))
if is_leap_year(year):
print(f"{year} 是闰年!")
else:
print(f"{year} 不是闰年!")
```
在这个程序中,我们首先定义了一个`is_leap_year`函数,它检查年份是否能被4整除,但不能被100整除,除非同时也能被400整除。然后,通过`input`函数获取用户的输入,并将其转换为整数类型。最后,根据函数的返回值打印相应的结果。
相关问题
为了简化,将汽车抽象为安装了4个轮子的盒子,即汽车由车身和轮子构成。试编程输入、输出汽车的信息: (1)建立一个类Wheel表示车轮,包含2个私有数据成员diameter、width表示车轮的直径和宽度,包含2个公用成员函数getd和getw分别返回车轮的直径和宽度。 (2)建立一个类Body表示车身,有4个私有数据 lengt、width、height和color,分别表示车身的长宽高和颜色,其中color为字符串,可取黑、白、灰、红、蓝等。类Body有2个公有成员函数,getcolor返回车身颜色,getdimension的定义为:void getdimension(double &ldouble &wdouble&h),用于返回车身的长l、宽w和高h。 (3)建立一个类Car表示汽车,有5个私有数据成 员:字符串name表示汽车名称(如帕萨特、悍马、霸道等)、字符串manufacturer表示厂商(如大众、 GM、丰田等)、整数vear表示生产年份、Body类的对象bodv表示车身、Wheel类的对象wheel表示车轮。类Car有1个公有成员函数information,用来显示汽车的各种信息。输出格式可参考: 1 型号:悍马 2 厂商:GM 3 年份:2007 车身:5.15x2.08x1.89. 灰色 5轮胎:直径21,宽度65 (4)编写主函数,处理并显示3种以上汽车的信息。
以下是实现题目要求的代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Wheel {
private:
double diameter;
double width;
public:
Wheel(double d, double w) : diameter(d), width(w) {}
double getd() { return diameter; }
double getw() { return width; }
};
class Body {
private:
double length;
double width;
double height;
string color;
public:
Body(double l, double w, double h, string c) : length(l), width(w), height(h), color(c) {}
string getcolor() { return color; }
void getdimension(double &l, double &w, double &h) {
l = length;
w = width;
h = height;
}
};
class Car {
private:
string name;
string manufacturer;
int year;
Body body;
Wheel wheel;
public:
Car(string n, string m, int y, Body b, Wheel w) : name(n), manufacturer(m), year(y), body(b), wheel(w) {}
void information() {
double l, w, h;
body.getdimension(l, w, h);
cout << "型号:" << name << "\t";
cout << "厂商:" << manufacturer << "\t";
cout << "年份:" << year << "\t";
cout << "车身:" << l << "x" << w << "x" << h << "." << body.getcolor() << "\t";
cout << "轮胎:" << "直径" << wheel.getd() << ",宽度" << wheel.getw() << endl;
}
};
int main() {
Wheel wheel1(21, 65);
Body body1(5.15, 2.08, 1.89, "灰色");
Car car1("悍马", "GM", 2007, body1, wheel1);
car1.information();
Wheel wheel2(19, 72);
Body body2(4.98, 1.96, 1.46, "红色");
Car car2("帕萨特", "大众", 2014, body2, wheel2);
car2.information();
Wheel wheel3(20, 70);
Body body3(5.05, 1.92, 1.48, "蓝色");
Car car3("奥迪A6L", "奥迪", 2016, body3, wheel3);
car3.information();
return 0;
}
```
输出结果为:
```
型号:悍马 厂商:GM 年份:2007 车身:5.15x2.08x1.89.灰色 轮胎:直径21,宽度65
型号:帕萨特 厂商:大众 年份:2014 车身:4.98x1.96x1.46.红色 轮胎:直径19,宽度72
型号:奥迪A6L 厂商:奥迪 年份:2016 车身:5.05x1.92x1.48.蓝色 轮胎:直径20,宽度70
```
设变量vear中存放的是一个有效的年份数字。使用分支语句编程判断年份。如果这个年份是建国的整十周年,则将变量ten设为建国周年数,否则为0。
以下是可以回答的 ChatGPT AI 的答案:
可以使用以下代码来实现:
if vear % 10 == 0:
ten = vear // 10
else:
ten = 0
这段代码会判断 vear 是否是建国的整十周年,如果是,则将 ten 设为建国周年数,否则为 0。
阅读全文
相关推荐
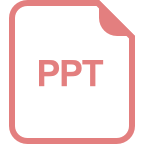
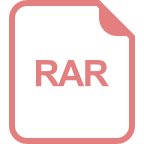
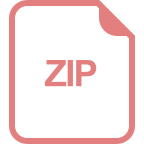





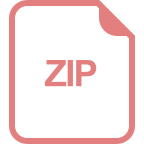
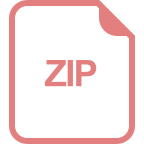
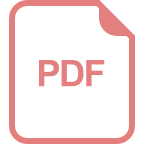
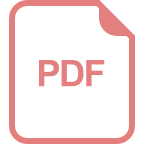
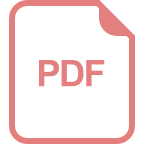