opengl中点画圆和椭圆代码
时间: 2024-11-04 09:15:11 浏览: 17
在OpenGL中绘制圆和椭圆,通常需要借助GLUT或其他图形库辅助,因为OpenGL本身并不直接提供这样的函数。你可以使用OpenGL的顶点着色器(v-shader)和片段着色器(f-shader)来绘制自定义几何形状。
绘制圆形的基本步骤是:
1. 定义一个圆心坐标、半径以及扇区数(用于细分圆弧)。
2. 创建两个三角形环,一个是顺时针,另一个是逆时针,通过glBegin(GL_LINES)连接顶点。
3. 使用 glVertex3f(x, y, 0) 或 glVertex3fv() 来设置每个顶点的坐标。
4. 使用glEnd() 结束顶点定义。
5. 在着色器中,根据输入的坐标数据渲染颜色。
对于椭圆,可以将其近似为由多个半径不等的圆组成。一种常见的做法是计算出足够精确的切线段,并将它们组合成椭圆的轮廓。
下面是一个简单的伪代码示例,实际操作中还需要编写完整的着色器和顶点数组等:
```cpp
// Pseudo-code
void drawCircle(float centerX, float centerY, float radius, int sectors) {
glBegin(GL_TRIANGLE_FAN);
for (int i = 0; i <= sectors; ++i) {
float angle = i * (2.0f * M_PI / sectors);
glVertex3f(centerX + radius * cos(angle), centerY + radius * sin(angle), 0);
}
glEnd();
}
void drawEllipse(float centerX, float centerY, float majorRadius, float minorRadius, int sectors) {
// Approximate ellipse with multiple circles
float step = 2.0f * M_PI / sectors;
for (float a = -M_PI; a < M_PI; a += step) {
float x = centerX + majorRadius * cos(a);
float y = centerY + minorRadius * sin(a);
drawCircle(x, y, minorRadius, sectors); // Draw minor circle segment
}
}
```
阅读全文
相关推荐
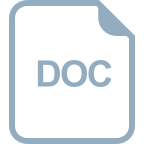
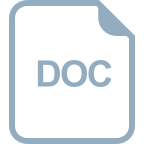
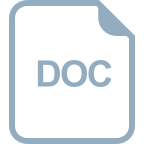
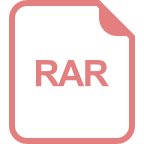
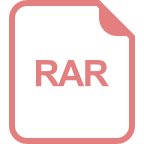
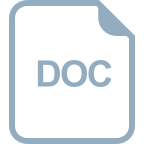
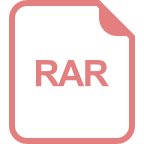
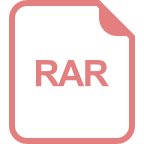
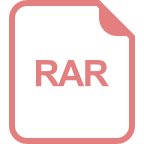
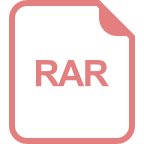
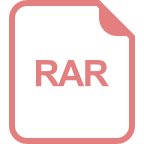
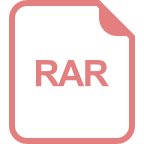
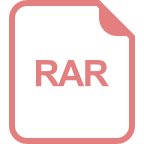
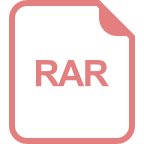
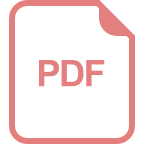
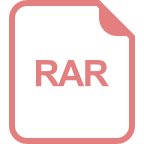
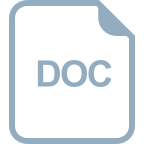
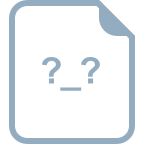
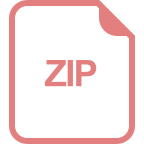