UnityWebRequest.Post和PostWWWForm各自的用法
时间: 2024-10-12 11:05:13 浏览: 24
UnityWebRequest.Post 和 PostWWWForm 都是在 Unity 游戏引擎中用于网络请求的工具,主要用于发送 POST 请求。
1. UnityWebRequest.Post:
- `UnityWebRequest` 是 Unity 的内置 API,提供了一个低级别的、线程安全的方式来发起 HTTP 请求。`UnityWebRequest.Post(url, data)` 这个方法用于发送 POST 请求,其中 `url` 是你要请求的服务器地址,`data` 是要发送的数据(通常是一个 JSON 字符串,可以用 `JsonUtility.ToJson()` 转换)。你需要设置好异步任务,并通过 `await` 关键字等待其完成,然后检查响应状态是否成功。
```csharp
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using System.Net;
public class NetworkExample : MonoBehaviour
{
public Button sendButton;
private async void SendPOSTRequest()
{
UnityWebRequest www = UnityWebRequest.Post("https://api.example.com/data", textData);
www.Send();
await www WaitUntilComplete();
if (www.isNetworkError || www.isHttpError)
Debug.LogError(www.error);
else
Debug.Log(www.downloadHandler.text);
}
}
```
2. PostWWWForm:
- `PostWWWForm` 则来自于第三方库 `WWWForm`,这个库的功能更强大,支持上传文件和表单数据。它创建了一个 `WWWForm` 对象,你可以添加键值对到其中,然后传递给 `WWW` 类的 `Post` 方法。`WWW` 类会处理发送和解析返回结果的过程。
```csharp
using UnityEngine;
using System.IO;
public class NetworkExample : MonoBehaviour
{
public Button sendButton;
public InputField usernameInput;
public FileUploader fileUploader;
private void SendPOSTRequest()
{
WWWForm form = new WWWForm();
form.AddField("username", usernameInput.text);
if (fileUploader != null && fileUploader.FileToUpload != null)
form.AddFileData("file", fileUploader.FileToUpload);
WWW www = new WWW("https://api.example.com/upload", form);
yield return www;
if (!string.IsNullOrEmpty(www.error))
Debug.LogError(www.error);
else
Debug.Log("Response: " + www.text);
}
}
```
阅读全文
相关推荐
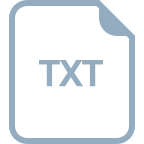
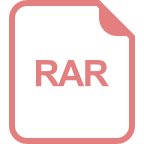







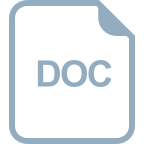








