java 图片指定长宽完整代码
时间: 2024-10-16 21:22:18 浏览: 21
在Java中,如果你想要加载图片并设置特定的宽度和高度,通常会使用`BufferedImage`类以及`ImageIO`来操作。以下是一个简单的示例,展示了如何创建一个新的图像,保持原始比例,然后按照指定大小缩放:
```java
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class ImageResizeExample {
public static BufferedImage resizeImage(String srcPath, int targetWidth, int targetHeight) {
File imgFile = new File(srcPath);
try {
BufferedImage originalImg = ImageIO.read(imgFile);
int width = originalImg.getWidth();
int height = originalImg.getHeight();
// 创建新的BufferedImage,并设置透明背景
BufferedImage resizedImg = new BufferedImage(targetWidth, targetHeight, BufferedImage.TYPE_INT_ARGB);
// 如果原始图像比目标尺寸大,按比例缩放
if (width > height && width > targetWidth || height > targetHeight) {
double scaleRatio = Math.min((double)targetWidth / width, (double)targetHeight / height);
int newWidth = (int)(width * scaleRatio);
int newHeight = (int)(height * scaleRatio);
// 使用AffineTransform进行等比例缩放
AffineTransform at = new AffineTransform();
at.scale(scaleRatio, scaleRatio);
at.translate(-(newWidth - targetWidth) / 2, -(newHeight - targetHeight) / 2);
Graphics2D g2d = resizedImg.createGraphics();
g2d.drawImage(originalImg, at, null);
g2d.dispose();
} else { // 否则,直接复制
resizedImg.getGraphics().drawImage(originalImg, 0, 0, targetWidth, targetHeight, null);
}
return resizedImg;
} catch (Exception e) {
System.out.println("Error reading or resizing image: " + e.getMessage());
return null; // 或者处理异常
}
}
public static void main(String[] args) {
String srcPath = "path_to_your_image.jpg";
int targetWidth = 500;
int targetHeight = 500;
BufferedImage resizedImg = resizeImage(srcPath, targetWidth, targetHeight);
if (resizedImg != null) {
// 现在你可以保存、显示或做其他操作
save(resizedImg, "resized_" + srcPath);
// OR
display(resizedImg);
}
}
// 保存图片到文件的方法
private static void save(BufferedImage img, String filePath) {
try {
ImageIO.write(img, "jpg", new File(filePath));
} catch (IOException e) {
System.out.println("Error saving the image: " + e.getMessage());
}
}
// 显示图片的方法(仅作示例,实际应用可能需要窗体或Swing)
private static void display(BufferedImage img) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JLabel label = new JLabel(new ImageIcon(img));
frame.add(label);
frame.pack();
frame.setVisible(true);
}
}
```
阅读全文
相关推荐
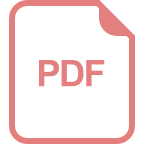
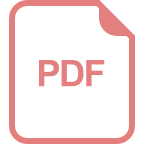
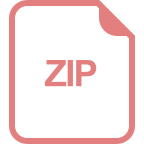















