请用python实现二分图的最大匹配算法。还需要用matplotlib.pyplot来实现可视化
时间: 2024-10-15 19:12:30 浏览: 36
在Python中,我们可以利用`networkx`库来创建二分图并求解最大匹配。然后结合`matplotlib.pyplot`进行可视化。这里是一个使用`networkx`库的Kuhn-Munkres算法(也称匈牙利算法)求解最大匹配的例子,同时也展示如何用`plt`绘制匹配结果:
```python
import networkx as nx
import matplotlib.pyplot as plt
# 创建二分图
def create_bipartite_graph():
G = nx.Graph()
# 添加源节点和目标节点,例如源节点为A-Z,目标节点为1-26
for letter in 'ABCDEFGHIJKLMNOPQRSTUVWXYZ':
G.add_node(letter)
for number in range(1, 27):
G.add_node(str(number))
# 添加一些随机边连接源和目标节点
edges = [(letter, str(number)) for letter, number in zip('ABCD', [1, 3, 4, 8])]
G.add_edges_from(edges)
return G
# Kuhn-Munkres算法求最大匹配
def hungarian_algorithm(graph):
matching = nx.max_weight_matching(graph, maxcardinality=True)
unmatched = list(set(graph.nodes) - set(matching.keys()))
return matching, unmatched
# 实现并绘制匹配结果
def visualize_matching(G, matching):
pos = {node: (0, i) if node.isupper() else (1, i) for i, node in enumerate(G)}
nx.draw_networkx_nodes(G, pos, nodelist=matching.keys(), node_color='r')
nx.draw_networkx_nodes(G, pos, nodelist=list(set(G)-matching.keys()), node_color='g')
nx.draw_networkx_edges(G, pos)
plt.title("Maximal Matching")
plt.show()
# 主程序
G = create_bipartite_graph()
matching, unmatched = hungarian_algorithm(G)
visualize_matching(G, matching)
```
这个代码首先创建了一个简单的二分图,然后使用Kuhn-Munkres算法找到最大匹配,并最后用`matplotlib`展示了匹配后的图形,红色节点表示已匹配,绿色节点表示未匹配。
阅读全文
相关推荐
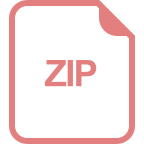
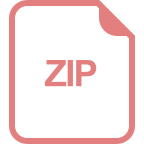
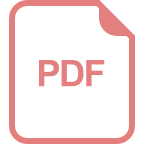




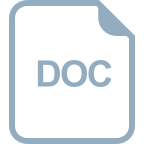




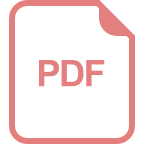
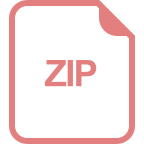
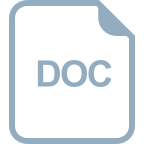
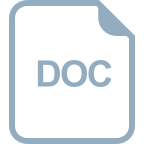



