设顺序表的类型为 sequenlist,请编写算法实现以下功能,输出顺序表中最大的数据元素及其所在位置用类 c 语言
时间: 2024-09-13 21:05:51 浏览: 96
为了在C语言中实现顺序表(sequenlist)中找到最大数据元素及其位置,我们可以创建一个结构体来表示顺序表的节点,并编写一个`SequenList`类来包含查找最大元素的方法。这里是一个简单的示例:
```c
// 定义顺序表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 定义顺序表类
typedef struct SequenList {
Node* head;
int size;
} SequenList;
// 初始化顺序表
void initSequenList(SequenList* seq) {
seq->head = NULL;
seq->size = 0;
}
// 插入节点到顺序表
void insert(SequenList* seq, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = seq->head;
seq->head = newNode;
seq->size++;
}
// 找到顺序表中的最大值和其位置
void findMaxAndPosition(SequenList* seq, int* maxIndex, int* maxValue) {
*maxValue = seq->head->data;
*maxIndex = 0;
Node* current = seq->head;
while (current != NULL) {
if (current->data > *maxValue) {
*maxValue = current->data;
*maxIndex = current->data - seq->head->data + 1; // 注意索引从1开始计数
}
current = current->next;
}
}
// 主函数
int main() {
SequenList seq;
int size, data, maxIndex, maxValue;
initSequenList(&seq);
printf("请输入顺序表的大小:");
scanf("%d", &size);
for (int i = 0; i < size; i++) {
printf("请输入顺序表的第%d个元素:", i+1);
scanf("%d", &data);
insert(&seq, data);
}
maxIndex = 0;
maxValue = 0;
findMaxAndPosition(&seq, &maxIndex, &maxValue);
printf("最大值为:%d,它在位置 %d。\n", maxValue, maxIndex + 1); // 注意索引加1
return 0;
}
```
阅读全文
相关推荐
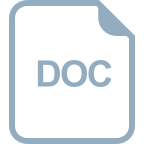
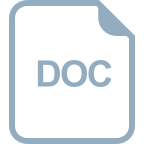
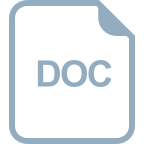
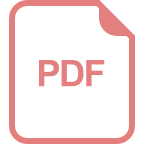

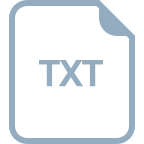
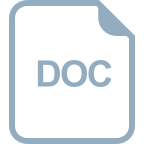
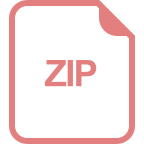
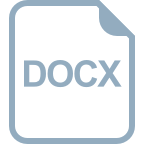
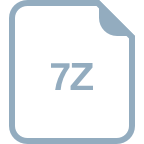
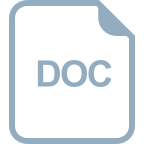
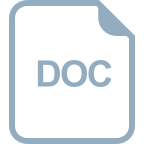
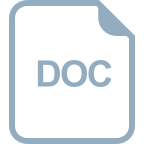
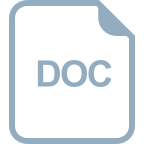
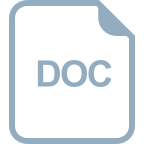
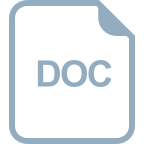

