solidity uint转string
时间: 2023-10-24 10:58:28 浏览: 291
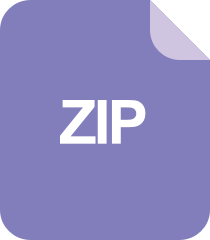
solidity
在 Solidity 中,将 uint 类型转换为 string 类型是一项相对复杂的任务,因为 Solidity 并没有提供直接的方法来实现此转换。但是,您可以使用以下方法之一来完成此任务:
方法一:使用字符串操作库(如 `solidity-stringutils`)
1. 在您的 Solidity 代码中导入 `solidity-stringutils` 库。
2. 使用库中提供的函数 `uintToString` 将 uint 类型转换为字符串类型。
下面是一个示例代码:
```solidity
pragma solidity ^0.8.0;
import "github.com/Arachnid/solidity-stringutils/strings.sol";
contract UintToStringExample {
using strings for *;
function uintToString(uint256 value) public pure returns (string memory) {
if (value == 0) {
return "0";
}
uint256 temp = value;
uint256 digits;
while (temp != 0) {
digits++;
temp /= 10;
}
strings.slice memory buffer = new strings.slice(digits);
temp = value;
while (temp != 0) {
buffer.push(uint8(temp % 10 + 48));
temp /= 10;
}
return buffer.toString();
}
}
```
方法二:手动实现转换
如果您不想使用外部库,您也可以手动实现将 uint 转换为 string 的转换函数。这需要一些字符串操作和数学计算。以下是一个简单的示例:
```solidity
pragma solidity ^0.8.0;
contract UintToStringExample {
function uintToString(uint256 value) public pure returns (string memory) {
if (value == 0) {
return "0";
}
uint256 temp = value;
uint256 digits;
while (temp != 0) {
digits++;
temp /= 10;
}
bytes memory buffer = new bytes(digits);
temp = value;
while (temp != 0) {
uint256 remainder = temp % 10;
temp /= 10;
buffer[--digits] = bytes1(uint8(48 + remainder));
}
return string(buffer);
}
}
```
这些示例代码可以将 uint 类型的变量转换为对应的字符串。请注意,在实际使用中,您可能需要对输入值进行额外的验证和处理,以确保转换结果正确无误。
阅读全文
相关推荐
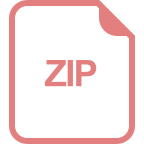
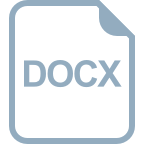















