在visual c++中分别实现直线段DDA算法、中点算法、Bresenham算法,要求: (1)三个算法写在一个程序中; (2)三个算法分别输入不同的起始点和终止点;
时间: 2024-09-06 16:06:20 浏览: 109
在Visual C++中实现直线段的三种绘制算法——DDA(Double Dithering Algorithm)、中点法(Midpoint Algorithm) 和 Bresenham算法,你可以创建一个包含三个函数的程序,每个函数处理一种算法。这里是一个简单的例子,假设我们已经有了`Point`结构体表示二维坐标,并有`drawLine()`函数作为公共接口,接收起点和终点。
```cpp
#include <iostream>
using namespace std;
struct Point {
int x;
int y;
};
// 双线性插值算法 (DDA)
void drawLine_DDA(Point start, Point end) {
double dx = end.x - start.x;
double dy = end.y - start.y;
int steep = abs(dy) > abs(dx);
if (steep) {
swap(start.x, start.y);
swap(end.x, end.y);
}
double err = dx / 2.0; // 初始化误差
for (int x = start.x; x <= end.x; ++x) {
if (steep) {
cout << start.y + floor(err) << " ";
start.y += floor(dy);
} else {
cout << start.x << " ";
start.x += 1;
}
err -= abs(dy);
if (err < 0) {
err += dx;
}
}
cout << endl;
}
// 中点法
void drawLine_Midpoint(Point start, Point end) {
if (start.x == end.x) { // 平行于y轴
for (int y = start.y; y <= end.y; ++y) {
cout << start.x << " ";
}
} else if (start.y == end.y) { // 平行于x轴
for (int x = start.x; x <= end.x; ++x) {
cout << x << " ";
}
} else {
int dx = end.x - start.x;
int dy = end.y - start.y;
int error = 2 * dy - dx;
while (start.x != end.x) {
cout << start.x << " ";
start.x++;
if (error >= 0) {
start.y++;
error += 2 * dx;
} else {
error -= 2 * dy;
}
}
cout << start.x << " "; // 输出终点
}
cout << endl;
}
// Bresenham算法
void drawLine_Bresenham(Point start, Point end) {
int dx = abs(end.x - start.x);
int dy = abs(end.y - start.y);
bool swapped = false;
if (start.x > end.x) {
swap(start.x, end.x);
swapped = true;
}
if (start.y > end.y) {
swap(start.y, end.y);
}
int error = dx - dy;
for (int x = start.x; x <= end.x; ++x) {
if (!swapped) {
cout << x << " ";
} else {
cout << start.y << " ";
}
error += 2 * dy;
if (error >= dx) {
error -= 2 * dx;
start.y++;
}
}
cout << endl;
}
int main() {
Point start, end;
cout << "请输入起始点(x,y): ";
cin >> start.x >> start.y;
cout << "请输入终止点(x,y): ";
cin >> end.x >> end.y;
cout << "DDA算法: ";
drawLine_DDA(start, end);
cout << "中点算法: ";
drawLine_Midpoint(start, end);
cout << "Bresenham算法: ";
drawLine_Bresenham(start, end);
return 0;
}
```
阅读全文
相关推荐



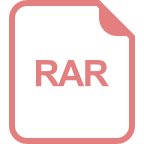
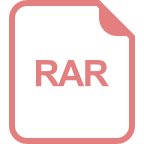











