俄罗斯方块Python
时间: 2023-11-29 18:43:02 浏览: 101
俄罗斯方块Python是一款基于Python语言编写的俄罗斯方块游戏。该游戏的目标是通过移动、旋转和放置不同形状的方块,使它们在底部形成完整的行,以便消除这些行并获得分数。该游戏具有基础页面界面动起来生成、移动、固定、变形消除与得分等功能。你可以通过引用中提供的资源下载源代码、打包好的可执行文件和文档报告,也可以通过引用中提供的演示效果下载地址查看该游戏的运行效果。
相关问题
俄罗斯方块python
俄罗斯方块是一个非常经典的游戏,它可以用Python编写。以下是一个简单的示例代码,可以让你开始构建你自己的俄罗斯方块游戏。
```python
import pygame
import random
# 定义方块的形状
tetrominoes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6, 6, 6]],
[[7, 7],
[7, 7]]
]
# 初始化游戏
def init_game():
global screen, clock, font, score, board, current_tetromino, next_tetromino
pygame.init()
screen = pygame.display.set_mode((240, 480))
pygame.display.set_caption('Tetris')
clock = pygame.time.Clock()
font = pygame.font.SysFont(None, 25)
score = 0
board = [[0 for _ in range(10)] for _ in range(20)]
current_tetromino = Tetromino()
next_tetromino = Tetromino()
# 定义方块类
class Tetromino:
def __init__(self):
self.x = 4
self.y = 0
self.shape = random.choice(tetrominoes)
self.color = random.randint(1, 7)
def rotate(self):
self.shape = [[self.shape[y][x] for y in range(len(self.shape))] for x in range(len(self.shape[0]) - 1, -1, -1)]
def move_left(self):
self.x -= 1
if self.collides():
self.x += 1
def move_right(self):
self.x += 1
if self.collides():
self.x -= 1
def move_down(self):
self.y += 1
if self.collides():
self.y -= 1
self.freeze()
def collides(self):
for y, row in enumerate(self.shape):
for x, cell in enumerate(row):
if cell and (self.y + y >= len(board) or self.x + x < 0 or self.x + x >= len(board[0]) or board[self.y + y][self.x + x]):
return True
return False
def freeze(self):
for y, row in enumerate(self.shape):
for x, cell in enumerate(row):
if cell:
board[self.y + y][self.x + x] = self.color
remove_rows()
new_tetromino()
# 绘制方块
def draw_block(x, y, color):
pygame.draw.rect(screen, (0, 0, 0), (x, y, 20, 20), 1)
pygame.draw.rect(screen, colors[color], (x + 1, y + 1, 18, 18))
# 绘制游戏区域
def draw_board():
for y, row in enumerate(board):
for x, cell in enumerate(row):
if cell:
draw_block(x * 20, y * 20, cell)
# 绘制得分
def draw_score():
score_text = font.render('Score: ' + str(score), True, (255, 255, 255))
screen.blit(score_text, (10, 460))
# 删除已经填满的行
def remove_rows():
global score
complete_rows = [row for row in range(len(board)) if all(board[row])]
for row in complete_rows:
del board[row]
board.insert(0, [0 for _ in range(10)])
score += len(complete_rows) ** 2
# 生成新的方块
def new_tetromino():
global current_tetromino, next_tetromino
current_tetromino = next_tetromino
next_tetromino = Tetromino()
# 主循环
def main_loop():
while True:
clock.tick(30)
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_tetromino.move_left()
if event.key == pygame.K_RIGHT:
current_tetromino.move_right()
if event.key == pygame.K_DOWN:
current_tetromino.move_down()
if event.key == pygame.K_UP:
current_tetromino.rotate()
screen.fill((0, 0, 0))
draw_board()
draw_score()
for y, row in enumerate(next_tetromino.shape):
for x, cell in enumerate(row):
if cell:
draw_block(x * 20 + 160, y * 20 + 320, next_tetromino.color)
for y, row in enumerate(current_tetromino.shape):
for x, cell in enumerate(row):
if cell:
draw_block((current_tetromino.x + x) * 20, (current_tetromino.y + y) * 20, current_tetromino.color)
pygame.display.update()
# 开始游戏
if __name__ == '__main__':
colors = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255), (255, 165, 0)]
init_game()
main_loop()
```
这段代码使用了Pygame库来处理游戏的图形和事件。它定义了一个方块类,用于创建和移动方块,并定义了一个主循环,在其中处理游戏逻辑并绘制游戏界面。您可以根据需要修改代码以改变游戏的行为和外观。
俄罗斯方块 python
俄罗斯方块是一款经典的游戏,而在Python中,我们可以使用PyGame库来编写一个简单的俄罗斯方块游戏。根据引用提供的信息,有人已经测试并成功地用Python3.6和Tkinter重新编译了俄罗斯方块的源代码,并进行了一些修改。
根据引用提供的教程,我们可以开始编写俄罗斯方块游戏的代码。这篇教程会重点关注游戏的逻辑,而不是PyGame库的内部原理。如果你不想阅读整篇文章,可以直接复制文末提供的代码。
在开始编写之前,需要做一些准备工作。首先,你需要安装Python 3。你可以从官方网站下载最新的Python版本。其次,你需要安装PyGame库。根据你使用的操作系统,打开命令提示符或终端,输入"pip install pygame"或"pip3 install pygame"来安装PyGame库。最后,你需要对Python有一些基本的了解。如果需要,可以参考其他博文来学习Python的基础知识。
通过阅读引用和引用提供的信息,你可以开始编写俄罗斯方块的Python代码,并根据引用提供的准备工作进行设置。这样你就可以开始享受自己编写的俄罗斯方块游戏了。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [俄罗斯方块python源码](https://download.csdn.net/download/finger2017/10270743)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [Python 写一个俄罗斯方块游戏](https://blog.csdn.net/weixin_46089319/article/details/106639305)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
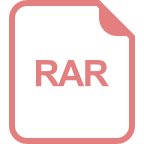
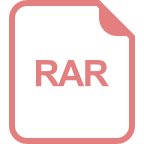




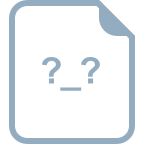
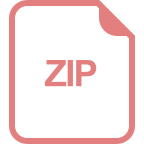
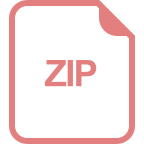
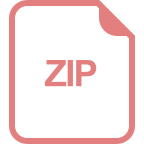