俄罗斯方块python原码
时间: 2023-10-12 12:17:50 浏览: 32
以下是一个简单的俄罗斯方块游戏的 Python 原码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 游戏区域大小
size = (320, 480)
screen = pygame.display.set_mode(size)
# 游戏区域颜色
bg_color = (255, 255, 255)
# 游戏区域边界大小
border_size = 20
# 方块大小
block_size = 20
# 方块移动速度
block_speed = 1
# 方块下落速度
fall_speed = 1
# 方块颜色
colors = [
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(0, 255, 255),
(255, 0, 255),
(128, 128, 128),
]
# 方块类型
blocks = {
0: [[1, 1, 1], [0, 1, 0]],
1: [[1, 1, 0], [0, 1, 1]],
2: [[0, 1, 1], [1, 1, 0]],
3: [[1, 1], [1, 1]],
4: [[1, 0, 0], [1, 1, 1]],
5: [[0, 0, 1], [1, 1, 1]],
6: [[1, 1, 1, 1]],
}
# 方块初始位置
block_x = 4
block_y = 0
# 随机生成方块
current_block = random.choice(list(blocks.keys()))
current_block_rotation = 0
current_block_color = random.choice(colors)
# 游戏区域数组
board = [[0 for x in range(10)] for y in range(20)]
# 循环更新游戏区域
done = False
while not done:
# 监听事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
# 左移方块
if all(board[block_y + i][block_x - 1] == 0 for i, row in enumerate(blocks[current_block][current_block_rotation])):
block_x -= 1
elif event.key == pygame.K_RIGHT:
# 右移方块
if all(board[block_y + i][block_x + len(blocks[current_block][current_block_rotation][0])] == 0 for i, row in enumerate(blocks[current_block][current_block_rotation])):
block_x += 1
elif event.key == pygame.K_UP:
# 旋转方块
new_rotation = (current_block_rotation + 1) % len(blocks[current_block])
if all(board[block_y + i][block_x + j] == 0 for i, row in enumerate(blocks[current_block][new_rotation]) for j, col in enumerate(row)):
current_block_rotation = new_rotation
elif event.key == pygame.K_DOWN:
# 快速下落方块
while all(board[block_y + i + 1][block_x + j] == 0 for i, row in enumerate(blocks[current_block][current_block_rotation]) for j, col in enumerate(row)):
block_y += 1
# 更新方块位置
if fall_speed % 10 == 0:
if all(board[block_y + i + 1][block_x + j] == 0 for i, row in enumerate(blocks[current_block][current_block_rotation]) for j, col in enumerate(row)):
block_y += 1
else:
# 方块落地
for i, row in enumerate(blocks[current_block][current_block_rotation]):
for j, col in enumerate(row):
if col == 1:
board[block_y + i][block_x + j] = current_block_color
# 生成新方块
current_block = random.choice(list(blocks.keys()))
current_block_rotation = 0
current_block_color = random.choice(colors)
block_x = 4
block_y = 0
fall_speed += 1
# 绘制游戏区域
screen.fill(bg_color)
pygame.draw.rect(screen, (0, 0, 0), pygame.Rect(border_size, border_size, block_size * 10, block_size * 20), 1)
for i, row in enumerate(board):
for j, col in enumerate(row):
if col != 0:
pygame.draw.rect(screen, col, pygame.Rect(border_size + j * block_size, border_size + i * block_size, block_size, block_size))
for i, row in enumerate(blocks[current_block][current_block_rotation]):
for j, col in enumerate(row):
if col == 1:
pygame.draw.rect(screen, current_block_color, pygame.Rect(border_size + (block_x + j) * block_size, border_size + (block_y + i) * block_size, block_size, block_size))
pygame.display.flip()
# 控制游戏速度
pygame.time.wait(50)
# 退出 Pygame
pygame.quit()
```
此代码是一个简单的实现,可以根据需要进行修改和扩展。
相关推荐
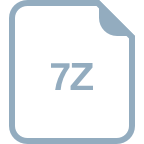














