用python写一个人脸识别系统代码,包括人脸检测、特征提取和人脸比对等步骤
时间: 2024-10-16 22:05:23 浏览: 36
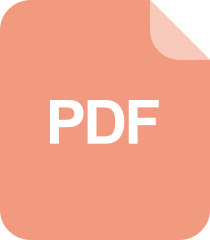
python调用OpenCV实现人脸识别功能
创建一个基本的人脸识别系统在Python中通常涉及多个库,如OpenCV(用于图像处理)、dlib(人脸检测和特征提取工具)以及face_recognition(人脸识别)。以下是一个简化版的示例,展示了如何实现这些步骤:
```python
# 首先安装必要的库
!pip install opencv-python dlib face_recognition
import cv2
import dlib
from PIL import Image
# 下载预训练的人脸识别模型
known_faces = []
known_names = []
def load_known_faces_from_db():
# 这里假设你有一个数据库,存储了人脸图片及其对应的名字
for name in known_names:
img = face_recognition.load_image_file(f"{name}.jpg") # 替换为你的文件路径
face_encoding = face_recognition.face_encodings(img)[0]
known_faces.append(face_encoding)
load_known_faces_from_db()
# 定义人脸检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 人脸识别主函数
def recognize_face(frame):
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector(gray)
for face in faces:
x1, y1, width, height = face.left(), face.top(), face.right() - face.left(), face.bottom() - face.top()
face_img = gray[y1:y1+height, x1:x1+width]
# 转换成PIL图像并调整大小
pil_img = Image.fromarray(face_img).resize((96, 96))
# 提取特征向量
face_encoding = face_recognition.face_encodings(pil_img)[0]
# 对比已知的人脸
results = face_recognition.compare_faces(known_faces, face_encoding)
if True in results:
# 如果找到匹配的人脸,返回名字
match_index = results.index(True)
print(f"Found match: {known_names[match_index]}")
else:
print("Unknown person")
# 获取摄像头输入,实时人脸识别
video_capture = cv2.VideoCapture(0) # 可以替换为视频文件路径
while True:
ret, frame = video_capture.read()
recognize_face(frame)
cv2.imshow('Face Recognition', frame)
key = cv2.waitKey(1)
# 关闭资源
video_capture.release()
cv2.destroyAllWindows()
```
这个例子只是一个基础框架,实际应用中你可能需要优化算法,比如增加性能提升的技术(如深度学习模型),并且要考虑如何持久化存储人脸信息以及提高系统的鲁棒性和错误处理。
阅读全文
相关推荐
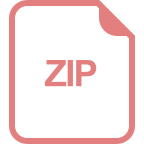
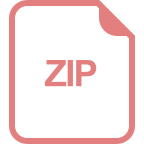

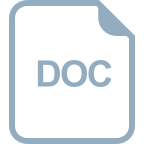
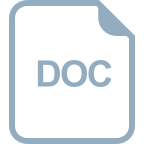
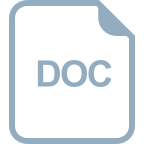
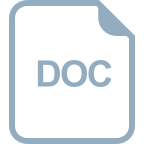
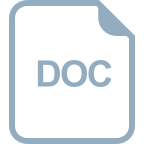
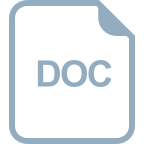
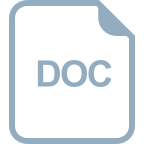
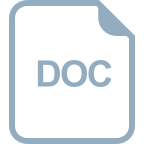






