定义一个复数类 Complex, 重载运算符“+”,使之能用于复数的加法运算。将运算符函数重载为非成员、非友元的普通函数。编写程序,求两个复数之和。
时间: 2024-11-05 20:24:58 浏览: 44
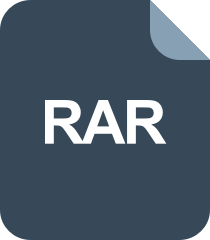
复数类加减法运算符重载成员函数

在C++中,我们可以创建一个名为Complex的类来表示复数,并重载"+"运算符,使其可以在两个复数之间进行加法运算。由于我们不想将其作为成员函数或友元函数处理,我们将定义一个静态成员函数。以下是步骤:
```cpp
#include <iostream>
using namespace std;
class Complex {
public:
// 构造函数
Complex(double real = 0.0, double imag = 0.0) : real(real), imag(imag) {}
// 获取复数的实部和虚部
double getReal() const { return real; }
double getImaginary() const { return imag; }
private:
double real;
double imag;
};
// 非成员、非友元的加法运算符函数
Complex operator+(const Complex& c1, const Complex& c2) {
Complex sum;
sum.real = c1.getReal() + c2.getReal();
sum.imag = c1.getImaginary() + c2.getImaginary();
return sum;
}
int main() {
Complex num1(3.0, 4.0);
Complex num2(5.0, -2.0);
Complex result = num1 + num2;
cout << "The sum of " << num1.getReal() << " + " << num1.getImaginary() << "i and "
<< num2.getReal() << " + " << num2.getImaginary() << "i is: "
<< result.getReal() << " + " << result.getImaginary() << "i\n";
return 0;
}
```
在这个例子中,`operator+`函数接受两个Complex类型的参数,计算它们的实部和虚部分别相加,然后返回一个新的复数,代表两个输入复数的和。
阅读全文
相关推荐
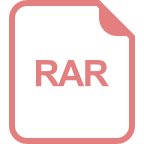
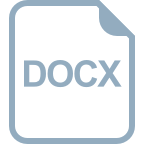















