ffmpeg读取MP4文件保存jpg
时间: 2024-01-13 19:20:09 浏览: 162
ffmpeg读取MP4文件并保存为jpg的主要函数部分如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libswscale/swscale.h>
int main(int argc, char *argv[]) {
AVFormatContext *pFormatCtx = NULL;
AVCodecContext *pCodecCtx = NULL;
AVCodec *pCodec = NULL;
AVFrame *pFrame = NULL;
AVPacket packet;
int videoStream = -1;
int frameFinished = 0;
int numBytes;
uint8_t *buffer = NULL;
struct SwsContext *sws_ctx = NULL;
int i;
// 打开输入文件
if (avformat_open_input(&pFormatCtx, argv[1], NULL, NULL) != 0) {
fprintf(stderr, "Could not open input file '%s'\n", argv[1]);
return -1;
}
// 获取流信息
if (avformat_find_stream_info(pFormatCtx, NULL) < 0) {
fprintf(stderr, "Could not find stream information\n");
return -1;
}
// 查找视频流
for (i = 0; i < pFormatCtx->nb_streams; i++) {
if (pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStream = i;
break;
}
}
if (videoStream == -1) {
fprintf(stderr, "Could not find video stream\n");
return -1;
}
// 获取视频解码器
pCodec = avcodec_find_decoder(pFormatCtx->streams[videoStream]->codecpar->codec_id);
if (pCodec == NULL) {
fprintf(stderr, "Unsupported codec\n");
return -1;
}
// 初始化解码器上下文
pCodecCtx = avcodec_alloc_context3(pCodec);
if (avcodec_parameters_to_context(pCodecCtx, pFormatCtx->streams[videoStream]->codecpar) < 0) {
fprintf(stderr, "Failed to copy codec parameters to decoder context\n");
return -1;
}
// 打开解码器
if (avcodec_open2(pCodecCtx, pCodec, NULL) < 0) {
fprintf(stderr, "Failed to open codec\n");
return -1;
}
// 分配帧内存
pFrame = av_frame_alloc();
if (pFrame == NULL) {
fprintf(stderr, "Failed to allocate frame\n");
return -1;
}
// 分配缓冲区
numBytes = av_image_get_buffer_size(AV_PIX_FMT_RGB24, pCodecCtx->width, pCodecCtx->height, 1);
buffer = (uint8_t *)av_malloc(numBytes * sizeof(uint8_t));
// 初始化SwsContext
sws_ctx = sws_getContext(pCodecCtx->width, pCodecCtx->height, pCodecCtx->pix_fmt,
pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_RGB24,
SWS_BILINEAR, NULL, NULL, NULL);
// 读取帧并保存为jpg
while (av_read_frame(pFormatCtx, &packet) >= 0) {
if (packet.stream_index == videoStream) {
avcodec_send_packet(pCodecCtx, &packet);
avcodec_receive_frame(pCodecCtx, pFrame);
// 转换像素格式
sws_scale(sws_ctx, (const uint8_t * const *)pFrame->data, pFrame->linesize, 0, pCodecCtx->height,
pFrame->data, pFrame->linesize);
// 保存为jpg文件
char filename[256];
sprintf(filename, "frame%d.jpg", i++);
FILE *file = fopen(filename, "wb");
fwrite(buffer, 1, numBytes, file);
fclose(file);
}
av_packet_unref(&packet);
}
// 释放资源
av_free(buffer);
av_frame_free(&pFrame);
avcodec_close(pCodecCtx);
avformat_close_input(&pFormatCtx);
return 0;
}
```
请注意,上述代码只是一个示例,可能需要根据实际情况进行适当的修改和调整。此外,还需要包含相应的头文件和链接相应的库文件。
阅读全文
相关推荐
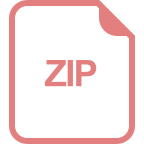
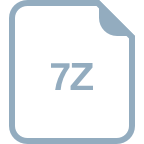
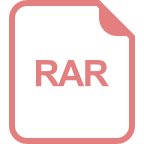
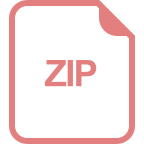
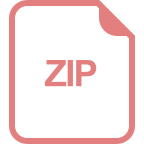
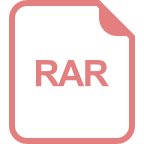
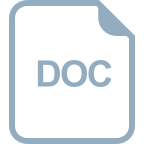

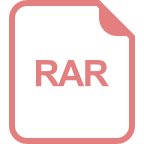
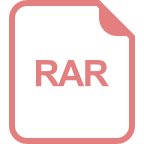
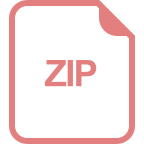
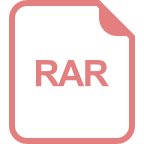
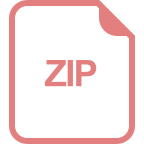
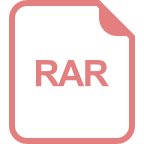


