汇编语言程序设计在内存中定义一个两位的BCD码,将这个两位数以十进制数形式显出来,并加上后缀D
时间: 2024-11-12 20:36:46 浏览: 36
汇编语言中,BCD(Binary Coded Decimal,二进制编码十进制)是一种用于存储十进制数字的传统方法,每个位用四位二进制来表示0-9这十个数字。如果你想在内存中定义一个两位BCD码并将其转换为十进制显示,并加上后缀"D",可以按照以下步骤操作:
1. **定义变量**: 首先,你需要分配两个字节的空间来保存两位BCD码。例如,在Intel x86汇编语言中,你可以用`DW`指令声明一个双字(word-wide,即两个字节)的存储空间。
```assembly
section .data
bcdCode DW ?
```
2. **加载BCD码**: 将实际的十进制数值转换为BCD码并存入该变量。假设我们要存储的十进制数是`num`,这个过程需要手动计算(因为汇编语言没有内置函数)。例如:
```assembly
mov ax, num ; Load the decimal number into AX register
lea bx, bcdCode ; Point to the BCD code location
; Convert each digit (low and high nibble)
and al, 0F ; AL = low nibble (0-9)
add al, 30h ; Add ASCII '0' value to get ASCII char for low nibble
stosb ; Store low nibble in memory
shl ax, 4 ; Shift high nibble left by 4 bits
and ah, 0F ; AH = high nibble (0-9)
add ah, 30h ; Same as above but for high nibble
stosb ; Store high nibble in memory
```
3. **转换和显示**: 从内存中取出BCD码,然后转换回十进制。这通常需要一些额外的操作,如减去ASCII偏移量并取整。这里仅示例代码的一部分,完整的转换过程可能更复杂。
```assembly
; Load two nibbles back into AX
lodsb
adc ax, 0 ; AX += high nibble (accounting for carry from low nibble)
lodsb
adc ax, 0 ; AX += low nibble (final result is in AX)
; Now AX holds the decimal value
push ax ; Save it on the stack or a register
push byte 'D' ; Push 'D' character for suffix
call printNumber ; Call your custom function to display the number
pop eax ; Restore the original number
section .text
printNumber:
; Your custom printing function here...
ret
```
4. **相关问题--**
1. 如何在汇编中处理多位的BCD码?
2. 汇编语言有没有现成函数可以直接转换BCD到十进制?
3. 如果要支持负数的BCD表示,应该如何修改上述代码?
阅读全文
相关推荐




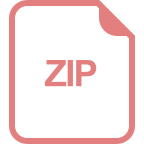













