C语言链表多项式相加代码
时间: 2024-04-21 20:19:52 浏览: 104
当然,我可以给你介绍一下C语言链表多项式相加的代码。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义多项式的节点结构
typedef struct Node {
int coefficient; // 系数
int exponent; // 指数
struct Node* next;
} Node;
// 创建一个新节点
Node* createNode(int coefficient, int exponent) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->coefficient = coefficient;
newNode->exponent = exponent;
newNode->next = NULL;
return newNode;
}
// 向多项式中插入一个节点
void insertNode(Node** poly, int coefficient, int exponent) {
Node* newNode = createNode(coefficient, exponent);
if (*poly == NULL) {
*poly = newNode;
} else {
Node* temp = *poly;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 打印多项式
void printPolynomial(Node* poly) {
Node* temp = poly;
while (temp != NULL) {
printf("%dx^%d ", temp->coefficient, temp->exponent);
if (temp->next != NULL) {
printf("+ ");
}
temp = temp->next;
}
printf("\n");
}
// 相加两个多项式
Node* addPolynomials(Node* poly1, Node* poly2) {
Node* result = NULL;
Node* temp1 = poly1;
Node* temp2 = poly2;
while (temp1 != NULL && temp2 != NULL) {
if (temp1->exponent > temp2->exponent) {
insertNode(&result, temp1->coefficient, temp1->exponent);
temp1 = temp1->next;
} else if (temp1->exponent < temp2->exponent) {
insertNode(&result, temp2->coefficient, temp2->exponent);
temp2 = temp2->next;
} else {
int sum = temp1->coefficient + temp2->coefficient;
if (sum != 0) {
insertNode(&result, sum, temp1->exponent);
}
temp1 = temp1->next;
temp2 = temp2->next;
}
}
// 将剩余的节点添加到结果中
while (temp1 != NULL) {
insertNode(&result, temp1->coefficient, temp1->exponent);
temp1 = temp1->next;
}
while (temp2 != NULL) {
insertNode(&result, temp2->coefficient, temp2->exponent);
temp2 = temp2->next;
}
return result;
}
int main() {
Node* poly1 = NULL;
Node* poly2 = NULL;
// 创建第一个多项式
insertNode(&poly1, 3, 4);
insertNode(&poly1, 2, 3);
insertNode(&poly1, 5, 1);
// 创建第二个多项式
insertNode(&poly2, 4, 3);
insertNode(&poly2, 1, 2);
insertNode(&poly2, 2, 0);
printf("第一个多项式:");
printPolynomial(poly1);
printf("第二个多项式:");
printPolynomial(poly2);
Node* result = addPolynomials(poly1, poly2);
printf("相加后的多项式:");
printPolynomial(result);
return 0;
}
```
这段代码实现了链表表示的多项式相加。首先定义了一个多项式的节点结构,包含系数和指数,并且有一个指向下一个节点的指针。然后通过`createNode`函数创建一个新节点,`insertNode`函数向多项式中插入一个节点,`printPolynomial`函数打印多项式。
最后,`addPolynomials`函数实现了两个多项式相加的功能。它遍历两个多项式的节点,根据指数的大小进行比较,将节点插入到结果多项式中。如果指数相同,则将系数相加,如果和不为0,则插入到结果多项式中。
在`main`函数中,创建了两个多项式,并调用`addPolynomials`函数进行相加操作,最后打印出相加后的多项式。
阅读全文
相关推荐
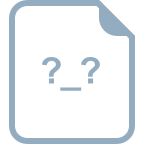


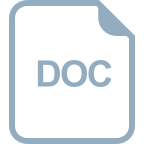
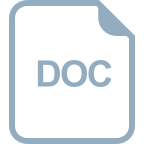
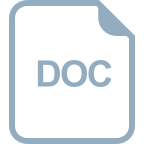
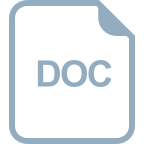



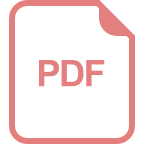
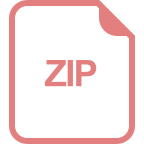



