C语言用链表实现一元多项式相加
时间: 2023-05-29 09:01:57 浏览: 91
#include <stdio.h>
#include <stdlib.h>
struct node { // 定义节点结构体
int coef; // 系数
int exp; // 指数
struct node *next; // 指向下一个节点的指针
};
typedef struct node Node;
Node* create_node(int coef, int exp) { // 创建一个节点
Node *new = (Node*)malloc(sizeof(Node));
new->coef = coef;
new->exp = exp;
new->next = NULL;
return new;
}
Node* add_poly(Node *poly1, Node *poly2) { // 两个多项式相加
Node *head = create_node(0, 0); // 头结点,作为新的多项式的起点
Node *p1 = poly1, *p2 = poly2, *p3 = head;
while (p1 != NULL && p2 != NULL) {
if (p1->exp > p2->exp) { // 计算结果多项式的该项为第一个多项式的当前项
p3->next = create_node(p1->coef, p1->exp);
p1 = p1->next;
} else if (p1->exp < p2->exp) { // 计算结果多项式的该项为第二个多项式的当前项
p3->next = create_node(p2->coef, p2->exp);
p2 = p2->next;
} else { // 计算结果多项式的该项为两个多项式的当前项之和,系数相加
p3->next = create_node(p1->coef + p2->coef, p1->exp);
p1 = p1->next;
p2 = p2->next;
}
p3 = p3->next;
}
// 处理没有计算过的多项式项
while (p1 != NULL) {
p3->next = create_node(p1->coef, p1->exp);
p1 = p1->next;
p3 = p3->next;
}
while (p2 != NULL) {
p3->next = create_node(p2->coef, p2->exp);
p2 = p2->next;
p3 = p3->next;
}
return head->next; // 返回新的多项式
}
void print_poly(Node *poly) { // 输出多项式
Node *p = poly;
while (p != NULL) {
printf("%dX^%d ", p->coef, p->exp);
if (p->next != NULL && p->next->coef > 0) {
printf("+ ");
}
p = p->next;
}
printf("\n");
}
int main() {
Node *poly1, *poly2, *result;
// 创建第一个多项式
poly1 = create_node(3, 5);
poly1->next = create_node(-2, 3);
poly1->next->next = create_node(1, 1);
// 创建第二个多项式
poly2 = create_node(2, 4);
poly2->next = create_node(-7, 3);
poly2->next->next = create_node(4, 1);
result = add_poly(poly1, poly2); // 两个多项式相加
printf("多项式1:");
print_poly(poly1); // 输出第一个多项式
printf("多项式2:");
print_poly(poly2); // 输出第二个多项式
printf("相加结果:");
print_poly(result); // 输出相加结果
// 释放内存
while (poly1 != NULL) {
Node *p = poly1;
poly1 = poly1->next;
free(p);
}
while (poly2 != NULL) {
Node *p = poly2;
poly2 = poly2->next;
free(p);
}
while (result != NULL) {
Node *p = result;
result = result->next;
free(p);
}
return 0;
}
相关推荐
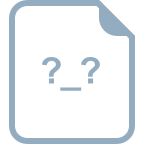














