C语言用链表实现多项式的加法
时间: 2023-07-11 07:04:04 浏览: 71
下面是 C 语言中用链表实现多项式加法的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义多项式结点
typedef struct PolyNode {
int coef; // 系数
int expon; // 指数
struct PolyNode* next;
} PolyNode, *PolyList;
// 多项式加法
PolyList PolyAdd(PolyList P1, PolyList P2)
{
PolyList front, rear, temp;
int sum;
// 初始化空结点
rear = (PolyList)malloc(sizeof(PolyNode));
front = rear;
while (P1 && P2) {
if (P1->expon == P2->expon) {
sum = P1->coef + P2->coef;
if (sum) {
temp = (PolyList)malloc(sizeof(PolyNode));
temp->coef = sum;
temp->expon = P1->expon;
rear->next = temp;
rear = temp;
}
P1 = P1->next;
P2 = P2->next;
} else if (P1->expon > P2->expon) {
temp = (PolyList)malloc(sizeof(PolyNode));
temp->coef = P1->coef;
temp->expon = P1->expon;
rear->next = temp;
rear = temp;
P1 = P1->next;
} else {
temp = (PolyList)malloc(sizeof(PolyNode));
temp->coef = P2->coef;
temp->expon = P2->expon;
rear->next = temp;
rear = temp;
P2 = P2->next;
}
}
// 将未处理完的结点添加到结果链表中
for (; P1; P1 = P1->next) {
temp = (PolyList)malloc(sizeof(PolyNode));
temp->coef = P1->coef;
temp->expon = P1->expon;
rear->next = temp;
rear = temp;
}
for (; P2; P2 = P2->next) {
temp = (PolyList)malloc(sizeof(PolyNode));
temp->coef = P2->coef;
temp->expon = P2->expon;
rear->next = temp;
rear = temp;
}
// 结束链表
rear->next = NULL;
// 删除空结点
temp = front;
front = front->next;
free(temp);
return front;
}
// 创建多项式
PolyList CreatePoly()
{
PolyList P, rear, temp;
int coef, expon;
// 初始化空结点
rear = (PolyList)malloc(sizeof(PolyNode));
P = rear;
// 输入多项式的系数和指数
while (scanf("%d %d", &coef, &expon) == 2) {
if (!coef || !expon) {
break;
}
temp = (PolyList)malloc(sizeof(PolyNode));
temp->coef = coef;
temp->expon = expon;
rear->next = temp;
rear = temp;
}
// 结束链表
rear->next = NULL;
// 删除空结点
temp = P;
P = P->next;
free(temp);
return P;
}
// 打印多项式
void PrintPoly(PolyList P)
{
if (!P) {
printf("0 0\n");
return;
}
for (; P; P = P->next) {
printf("%d %d", P->coef, P->expon);
if (P->next) {
printf(" ");
}
}
printf("\n");
}
int main()
{
PolyList P1, P2, PP;
// 创建多项式 P1 和 P2
printf("请输入多项式 P1:\n");
P1 = CreatePoly();
printf("请输入多项式 P2:\n");
P2 = CreatePoly();
// 求多项式 P1 和 P2 的和
PP = PolyAdd(P1, P2);
// 打印结果
printf("P1 + P2 = ");
PrintPoly(PP);
return 0;
}
```
以上代码中,首先定义了多项式结点 `PolyNode`,然后定义了多项式链表 `PolyList`,其中每个结点包含系数 `coef` 和指数 `expon` 两个成员,以及一个指向下一个结点的指针 `next`。
接着,定义了函数 `PolyAdd` 实现了多项式加法,它通过遍历两个多项式链表,将指数相同的项相加,并添加到结果链表中,最后返回结果链表。
函数 `CreatePoly` 用于创建多项式,它通过输入系数和指数,创建多项式链表并返回。
函数 `PrintPoly` 用于打印多项式,它遍历多项式链表并按格式输出。
在 `main` 函数中,先分别创建多项式 `P1` 和 `P2`,然后求它们的和 `PP`,最后输出结果。
相关推荐
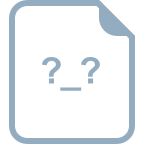
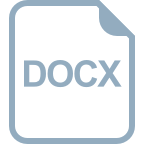














