请用C语言链表实现多项式的加减乘法
时间: 2023-08-15 10:17:54 浏览: 91
好的,我来回答你的问题。
链表是一种常用的数据结构,可以用来实现多项式的加减乘法。下面是 C 语言链表实现多项式的加减乘法的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 多项式结点结构体定义
typedef struct PolyNode {
int coef; // 系数
int expon; // 指数
struct PolyNode* next;
} PolyNode;
// 多项式结构体定义
typedef struct Poly {
PolyNode* head;
PolyNode* tail;
} Poly;
// 初始化多项式
void initPoly(Poly* poly) {
poly->head = NULL;
poly->tail = NULL;
}
// 添加多项式结点
void addNode(Poly* poly, int coef, int expon) {
PolyNode* node = (PolyNode*)malloc(sizeof(PolyNode));
node->coef = coef;
node->expon = expon;
node->next = NULL;
if (poly->tail == NULL) {
poly->head = node;
poly->tail = node;
} else {
poly->tail->next = node;
poly->tail = node;
}
}
// 输出多项式
void printPoly(Poly* poly) {
PolyNode* p = poly->head;
while (p != NULL) {
printf("%dx^%d", p->coef, p->expon);
if (p->next != NULL) {
printf(" + ");
}
p = p->next;
}
printf("\n");
}
// 释放多项式结点
void freePolyNode(PolyNode* node) {
if (node != NULL) {
freePolyNode(node->next);
free(node);
}
}
// 释放多项式
void freePoly(Poly* poly) {
freePolyNode(poly->head);
poly->head = NULL;
poly->tail = NULL;
}
// 多项式加法
Poly addPoly(Poly* poly1, Poly* poly2) {
Poly poly;
initPoly(&poly);
PolyNode* p1 = poly1->head;
PolyNode* p2 = poly2->head;
while (p1 != NULL && p2 != NULL) {
if (p1->expon > p2->expon) {
addNode(&poly, p1->coef, p1->expon);
p1 = p1->next;
} else if (p1->expon < p2->expon) {
addNode(&poly, p2->coef, p2->expon);
p2 = p2->next;
} else {
addNode(&poly, p1->coef + p2->coef, p1->expon);
p1 = p1->next;
p2 = p2->next;
}
}
while (p1 != NULL) {
addNode(&poly, p1->coef, p1->expon);
p1 = p1->next;
}
while (p2 != NULL) {
addNode(&poly, p2->coef, p2->expon);
p2 = p2->next;
}
return poly;
}
// 多项式减法
Poly subPoly(Poly* poly1, Poly* poly2) {
Poly poly;
initPoly(&poly);
PolyNode* p1 = poly1->head;
PolyNode* p2 = poly2->head;
while (p1 != NULL && p2 != NULL) {
if (p1->expon > p2->expon) {
addNode(&poly, p1->coef, p1->expon);
p1 = p1->next;
} else if (p1->expon < p2->expon) {
addNode(&poly, -p2->coef, p2->expon);
p2 = p2->next;
} else {
addNode(&poly, p1->coef - p2->coef, p1->expon);
p1 = p1->next;
p2 = p2->next;
}
}
while (p1 != NULL) {
addNode(&poly, p1->coef, p1->expon);
p1 = p1->next;
}
while (p2 != NULL) {
addNode(&poly, -p2->coef, p2->expon);
p2 = p2->next;
}
return poly;
}
// 多项式乘法
Poly mulPoly(Poly* poly1, Poly* poly2) {
Poly poly;
initPoly(&poly);
PolyNode* p1 = poly1->head;
PolyNode* p2 = poly2->head;
while (p1 != NULL) {
while (p2 != NULL) {
int coef = p1->coef * p2->coef;
int expon = p1->expon + p2->expon;
addNode(&poly, coef, expon);
p2 = p2->next;
}
p1 = p1->next;
p2 = poly2->head;
}
return poly;
}
// 测试
int main() {
Poly poly1, poly2, poly3, poly4;
initPoly(&poly1);
initPoly(&poly2);
initPoly(&poly3);
initPoly(&poly4);
addNode(&poly1, 3, 2);
addNode(&poly1, 4, 1);
addNode(&poly1, 5, 0);
addNode(&poly2, 2, 1);
addNode(&poly2, 1, 0);
printf("poly1: ");
printPoly(&poly1);
printf("poly2: ");
printPoly(&poly2);
poly3 = addPoly(&poly1, &poly2);
printf("poly1 + poly2: ");
printPoly(&poly3);
poly4 = subPoly(&poly1, &poly2);
printf("poly1 - poly2: ");
printPoly(&poly4);
Poly poly5 = mulPoly(&poly1, &poly2);
printf("poly1 * poly2: ");
printPoly(&poly5);
freePoly(&poly1);
freePoly(&poly2);
freePoly(&poly3);
freePoly(&poly4);
freePoly(&poly5);
return 0;
}
```
上述代码中,`PolyNode` 结构体表示多项式的一项,`Poly` 结构体表示多项式。`initPoly` 函数用于初始化多项式,`addNode` 函数用于添加多项式结点,`printPoly` 函数用于输出多项式,`freePolyNode` 函数用于释放多项式结点,`freePoly` 函数用于释放多项式,`addPoly` 函数实现多项式加法,`subPoly` 函数实现多项式减法,`mulPoly` 函数实现多项式乘法。
在主函数中,我们创建了两个多项式 `poly1` 和 `poly2`,分别为 3x^2 + 4x + 5 和 2x + 1。然后进行了多项式加减乘法的测试,并最终释放了多项式的内存。
注意,上述代码只是实现了多项式的加减乘法,还可以继续优化,例如优化多项式相加时的链表结构,以提高运行效率。
阅读全文
相关推荐

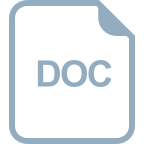
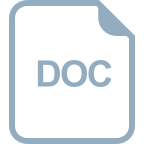
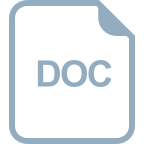
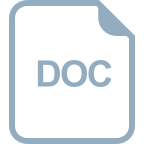
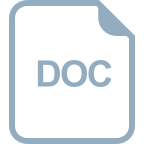
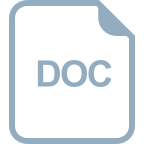
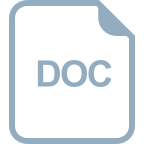
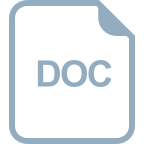
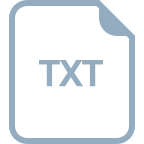
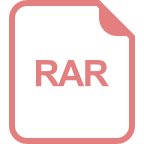
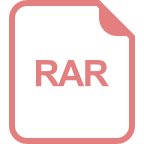
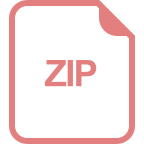
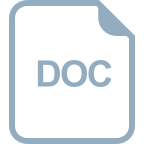
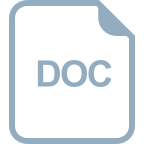