编写一个程序实现两个一元多项式相加的运算,c语言链表
时间: 2024-04-05 11:17:20 浏览: 32
#include<stdio.h>
#include<stdlib.h>
typedef struct Polynomial{
float coefficient;
int exponent;
struct Polynomial *next;
} Poly;
void createList(Poly **head, float coefficient, int exponent){
Poly *node = (Poly*)malloc(sizeof(Poly));
node->coefficient = coefficient;
node->exponent = exponent;
node->next = NULL;
if(*head == NULL){
*head = node;
} else{
Poly *p = *head;
while(p->next != NULL){
p = p->next;
}
p->next = node;
}
}
void input(Poly **head){
int n;
float coefficient;
int exponent;
printf("请输入多项式的项数:");
scanf("%d", &n);
for(int i=1; i<=n; i++){
printf("请输入第%d项的系数和指数:", i);
scanf("%f%d", &coefficient, &exponent);
createList(head, coefficient, exponent);
}
}
void output(Poly *head){
printf("多项式为:");
Poly *p = head;
while(p != NULL){
printf("%.2fx^%d", p->coefficient, p->exponent);
if(p->next != NULL){
printf("+");
}
p = p->next;
}
printf("\n");
}
void add(Poly *head1, Poly *head2, Poly **head3){
Poly *p = head1, *q = head2;
while(p != NULL && q != NULL){
if(p->exponent > q->exponent){
createList(head3, p->coefficient, p->exponent);
p = p->next;
} else if(p->exponent < q->exponent){
createList(head3, q->coefficient, q->exponent);
q = q->next;
} else{
createList(head3, p->coefficient+q->coefficient, p->exponent);
p = p->next;
q = q->next;
}
}
while(p != NULL){
createList(head3, p->coefficient, p->exponent);
p = p->next;
}
while(q != NULL){
createList(head3, q->coefficient, q->exponent);
q = q->next;
}
}
int main(){
Poly *head1=NULL, *head2=NULL, *head3=NULL;
printf("请输入第一个多项式:\n");
input(&head1);
printf("请输入第二个多项式:\n");
input(&head2);
output(head1);
output(head2);
add(head1, head2, &head3);
output(head3);
return 0;
}
相关推荐
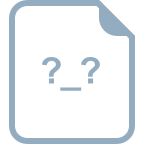














