用C语言实现【问题描述】编写一个程序实现两个一元多项式相加的运算。 【输入形式】从标准输入中读入两行以空格分隔的整数,每一行代表一个多项式,且该多项式中各项的系数均为0或正整数,最高幂次不超过50。对于多项式 anxn + an-1xn-1+ ... + a1x1 + a0x0 (n<50)的表示方法如下: an n an-1 n-1 ... a1 1 a0 0 即相邻两个整数分别表示表达式中一项的系数和指数。在输入中只出现系数不为0的项。 【输出形式】将运算结果输出到屏幕。将系数不为0的项按指数从高到低的顺序输出,每次输出其系数和指数,均以一个空格分隔。在行的末尾也输出一个空格符,并且最后要求换行。
时间: 2024-03-06 19:51:23 浏览: 122
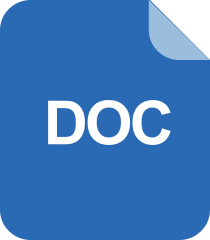
两个一元多项式相加的程序

以下是C语言的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义多项式节点结构体
typedef struct PolynomialNode {
int coef; // 系数
int exp; // 指数
struct PolynomialNode *next; // 指向下一个节点的指针
} PolynomialNode;
// 定义多项式链表结构体
typedef struct {
PolynomialNode *head; // 指向头节点的指针
PolynomialNode *tail; // 指向尾节点的指针
} PolynomialList;
// 初始化多项式链表
void initList(PolynomialList *list) {
list->head = NULL;
list->tail = NULL;
}
// 向多项式链表中插入一个节点
void insertNode(PolynomialList *list, int coef, int exp) {
PolynomialNode *node = (PolynomialNode *)malloc(sizeof(PolynomialNode));
node->coef = coef;
node->exp = exp;
node->next = NULL;
if (list->tail) {
list->tail->next = node;
list->tail = node;
} else {
list->head = node;
list->tail = node;
}
}
// 读入多项式
void read(PolynomialList *list) {
int coef, exp;
while (scanf("%d%d", &coef, &exp) == 2) {
insertNode(list, coef, exp);
}
}
// 输出多项式
void print(PolynomialList *list) {
int first_term = 1;
for (PolynomialNode *node = list->head; node; node = node->next) {
if (!first_term) {
printf(" ");
}
printf("%d %d", node->coef, node->exp);
first_term = 0;
}
printf(" \n");
}
// 多项式加法
PolynomialList add(PolynomialList *list1, PolynomialList *list2) {
PolynomialList result;
initList(&result);
PolynomialNode *node1 = list1->head;
PolynomialNode *node2 = list2->head;
while (node1 && node2) {
if (node1->exp == node2->exp) {
int coef = node1->coef + node2->coef;
if (coef != 0) {
insertNode(&result, coef, node1->exp);
}
node1 = node1->next;
node2 = node2->next;
} else if (node1->exp > node2->exp) {
insertNode(&result, node1->coef, node1->exp);
node1 = node1->next;
} else {
insertNode(&result, node2->coef, node2->exp);
node2 = node2->next;
}
}
while (node1) {
insertNode(&result, node1->coef, node1->exp);
node1 = node1->next;
}
while (node2) {
insertNode(&result, node2->coef, node2->exp);
node2 = node2->next;
}
return result;
}
int main() {
PolynomialList poly1, poly2, result;
initList(&poly1);
initList(&poly2);
read(&poly1);
read(&poly2);
result = add(&poly1, &poly2);
print(&result);
return 0;
}
```
在这个实现中,我们使用了链表来存储多项式,并通过函数来完成多项式的读入、输出和加法。多项式加法的实现过程中,我们需要遍历两个多项式链表,对于指数相同的项,将它们的系数相加并插入到结果链表中;对于指数不同的项,直接插入到结果链表中。最后,我们还需要处理其中一个多项式已经遍历完但另一个多项式还有剩余项的情况。
阅读全文
相关推荐
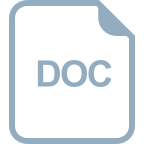
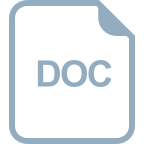








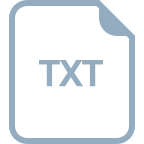
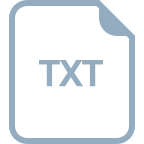
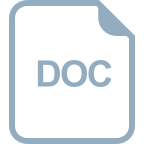
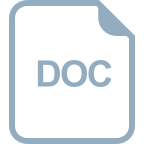
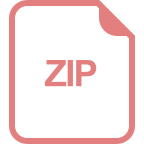
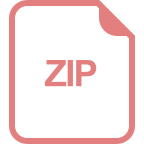
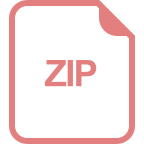
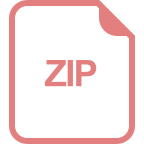