编程实现一元多项式的加法运算。第一个一元多项式A;第二个多项式B。以(0,0作为输入结束)C语言
时间: 2024-02-16 11:02:56 浏览: 54
以下是一元多项式的加法运算的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
/* 定义多项式的结构体 */
typedef struct PolyNode *Polynomial;
struct PolyNode {
int coef; /* 系数 */
int expon; /* 指数 */
Polynomial next; /* 指向下一项的指针 */
};
/* 多项式加法运算 */
Polynomial PolyAdd(Polynomial A, Polynomial B) {
Polynomial head, tail, temp;
head = (Polynomial) malloc(sizeof(struct PolyNode));
tail = head;
while (A && B) { /* A和B都非空 */
if (A->expon == B->expon) { /* 次数相等 */
int sum = A->coef + B->coef;
if (sum != 0) { /* 系数和不为0 */
temp = (Polynomial) malloc(sizeof(struct PolyNode));
temp->coef = sum;
temp->expon = A->expon;
tail->next = temp;
tail = temp;
}
A = A->next;
B = B->next;
} else if (A->expon > B->expon) { /* A的次数大于B的次数 */
temp = (Polynomial) malloc(sizeof(struct PolyNode));
temp->coef = A->coef;
temp->expon = A->expon;
tail->next = temp;
tail = temp;
A = A->next;
} else { /* A的次数小于B的次数 */
temp = (Polynomial) malloc(sizeof(struct PolyNode));
temp->coef = B->coef;
temp->expon = B->expon;
tail->next = temp;
tail = temp;
B = B->next;
}
}
/* 将剩余的项添加到结果多项式中 */
for (; A; A = A->next) {
temp = (Polynomial) malloc(sizeof(struct PolyNode));
temp->coef = A->coef;
temp->expon = A->expon;
tail->next = temp;
tail = temp;
}
for (; B; B = B->next) {
temp = (Polynomial) malloc(sizeof(struct PolyNode));
temp->coef = B->coef;
temp->expon = B->expon;
tail->next = temp;
tail = temp;
}
tail->next = NULL;
temp = head;
head = head->next;
free(temp); /* 释放头结点 */
return head;
}
/* 多项式读入 */
Polynomial ReadPoly() {
Polynomial head, tail, temp;
int n, c, e;
head = (Polynomial) malloc(sizeof(struct PolyNode));
head->next = NULL;
tail = head;
scanf("%d", &n); /* 读入项数 */
while (n--) {
scanf("%d %d", &c, &e);
temp = (Polynomial) malloc(sizeof(struct PolyNode));
temp->coef = c;
temp->expon = e;
tail->next = temp;
tail = temp;
}
tail->next = NULL;
temp = head;
head = head->next;
free(temp); /* 释放头结点 */
return head;
}
/* 多项式输出 */
void PrintPoly(Polynomial P) {
if (!P) {
printf("0 0\n");
return;
}
printf("%d %d", P->coef, P->expon);
P = P->next;
while (P) {
printf(" %d %d", P->coef, P->expon);
P = P->next;
}
printf("\n");
}
/* 主函数 */
int main() {
Polynomial A, B, C;
A = ReadPoly();
B = ReadPoly();
C = PolyAdd(A, B);
PrintPoly(C);
return 0;
}
```
其中,`PolyNode` 结构体表示一项多项式,`Polynomial` 则是指向 `PolyNode` 的指针类型。`PolyAdd` 函数实现了多项式的加法运算,`ReadPoly` 函数实现了多项式的读入,`PrintPoly` 函数实现了多项式的输出。在主函数中,先读入两个多项式,然后调用 `PolyAdd` 函数将它们相加,最后输出结果多项式。
相关推荐
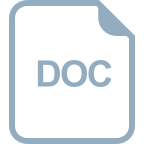
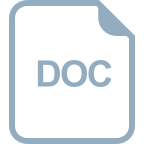
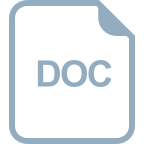
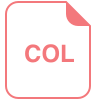
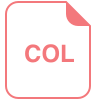
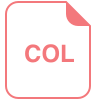
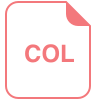
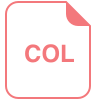






