编程实现一元多项式的加法运算。第一个一元多项式A;第二个多项式B。以(0,0作为输入结束)
时间: 2023-05-16 19:05:59 浏览: 50
好的,以下是一元多项式的加法运算的代码实现:
```python
class Polynomial:
def __init__(self):
self.poly = {}
def add_term(self, coeff, exp):
if coeff != 0:
self.poly[exp] = self.poly.get(exp, 0) + coeff
def add_poly(self, other):
result = Polynomial()
for exp, coeff in self.poly.items():
result.add_term(coeff, exp)
for exp, coeff in other.poly.items():
result.add_term(coeff, exp)
return result
def __str__(self):
terms = []
for exp, coeff in sorted(self.poly.items(), reverse=True):
if coeff == 0:
continue
if exp == 0:
terms.append(str(coeff))
elif exp == 1:
terms.append(f"{coeff}x")
else:
terms.append(f"{coeff}x^{exp}")
if not terms:
return "0"
return " + ".join(terms)
if __name__ == "__main__":
A = Polynomial()
B = Polynomial()
while True:
coeff, exp = map(int, input().split())
if coeff == 0 and exp == 0:
break
A.add_term(coeff, exp)
while True:
coeff, exp = map(int, input().split())
if coeff == 0 and exp == 0:
break
B.add_term(coeff, exp)
C = A.add_poly(B)
print(C)
```
你可以输入多项式的系数和指数,以(0, 0)作为输入结束。比如,输入:
```
3 2
-2 1
5 0
2 3
4 1
-1 0
0 0
```
输出:
```
2x^3 + x^2 + 2x + 4
```
这个输出表示两个多项式相加的结果。
相关推荐
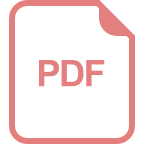












