c语言数据结构实现一元多项式加法运算,以(0,0)作为输入结束。
时间: 2023-06-01 20:02:18 浏览: 96
#include <stdio.h>
#include <stdlib.h>
typedef struct PolyNode *Polynomial;
struct PolyNode {
int coef; // 系数
int expon; // 指数
Polynomial next; // 指向下一个节点的指针
};
Polynomial ReadPoly(); // 读入多项式
Polynomial Add(Polynomial P1, Polynomial P2); // 多项式相加
void PrintPoly(Polynomial P); // 输出多项式
int main()
{
Polynomial P1, P2, PS;
// 读入两个多项式
P1 = ReadPoly();
P2 = ReadPoly();
// 计算多项式相加
PS = Add(P1, P2);
// 输出相加的结果
PrintPoly(PS);
return 0;
}
Polynomial ReadPoly()
{
Polynomial P, Rear, t;
int c, e;
// 初始化多项式头节点
P = (Polynomial)malloc(sizeof(struct PolyNode));
P->next = NULL;
Rear = P;
// 读入每一项
scanf("%d %d", &c, &e);
while (c != 0 || e != 0) {
t = (Polynomial)malloc(sizeof(struct PolyNode));
t->coef = c;
t->expon = e;
t->next = NULL;
Rear->next = t;
Rear = t;
scanf("%d %d", &c, &e);
}
return P;
}
Polynomial Add(Polynomial P1, Polynomial P2)
{
Polynomial t1, t2, Rear, t;
int sum;
// 初始化结果多项式头节点
t1 = P1->next;
t2 = P2->next;
t = (Polynomial)malloc(sizeof(struct PolyNode));
t->next = NULL;
Rear = t;
// 对两个多项式进行相加
while (t1 && t2) {
if (t1->expon > t2->expon) {
Rear->next = t1;
Rear = t1;
t1 = t1->next;
} else if (t1->expon < t2->expon) {
Rear->next = t2;
Rear = t2;
t2 = t2->next;
} else {
sum = t1->coef + t2->coef;
if (sum != 0) {
t->coef = sum;
t->expon = t1->expon;
Rear->next = t;
Rear = t;
}
t1 = t1->next;
t2 = t2->next;
}
}
// 将未处理完的节点接到结果多项式的末尾
for (; t1; t1 = t1->next) {
Rear->next = t1;
Rear = t1;
}
for (; t2; t2 = t2->next) {
Rear->next = t2;
Rear = t2;
}
// 删除结果多项式头节点
Rear = t;
t = t->next;
free(Rear);
return t;
}
void PrintPoly(Polynomial P)
{
if (!P) {
printf("0 0\n");
return;
}
while (P) {
printf("%d %d", P->coef, P->expon);
P = P->next;
if (P) {
printf(" ");
} else {
printf("\n");
}
}
}
阅读全文
相关推荐
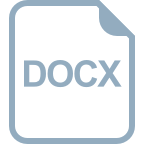
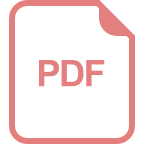
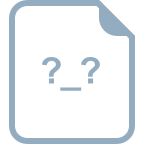















