c语言编程实现一元多项式的加法运算
时间: 2023-05-30 17:05:42 浏览: 139
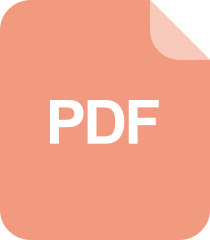
数据结构实验报告-一元多项式的加法运算.pdf
```
#include <stdio.h>
#include <stdlib.h>
typedef struct PolyNode *Polynomial;
struct PolyNode{
int coef; // 系数
int expon; // 指数
Polynomial link; // 下一项
};
void Attach(int c, int e, Polynomial *pRear){
Polynomial P;
// 新建节点
P = (Polynomial)malloc(sizeof(struct PolyNode));
P->coef = c;
P->expon = e;
P->link = NULL;
// 插入节点
(*pRear)->link = P;
*pRear = P;
}
Polynomial PolyAdd(Polynomial P1, Polynomial P2){
Polynomial P, Rear, t1, t2;
int sum;
// 新建头节点
P = (Polynomial)malloc(sizeof(struct PolyNode));
Rear = P;
t1 = P1->link;
t2 = P2->link;
while(t1 && t2){
if(t1->expon == t2->expon){ // 指数相等
sum = t1->coef + t2->coef;
if(sum) // 系数不为0
Attach(sum, t1->expon, &Rear);
t1 = t1->link;
t2 = t2->link;
}
else if(t1->expon > t2->expon){ // P1中指数较大
Attach(t1->coef, t1->expon, &Rear);
t1 = t1->link;
}
else{ // P2中指数较大
Attach(t2->coef, t2->expon, &Rear);
t2 = t2->link;
}
}
// 将未处理完的项接到结果多项式中
for(; t1; t1 = t1->link)
Attach(t1->coef, t1->expon, &Rear);
for(; t2; t2 = t2->link)
Attach(t2->coef, t2->expon, &Rear);
// 删除头节点
Rear->link = NULL;
P = P->link;
free(P1);
free(P2);
return P;
}
void PrintPoly(Polynomial P){
if(!P){
printf("0 0\n");
return;
}
while(P){
printf("%d %d", P->coef, P->expon);
P = P->link;
if(P)
printf(" ");
}
printf("\n");
}
int main(){
Polynomial P1, P2, PP, PS;
// 新建多项式P1
P1 = (Polynomial)malloc(sizeof(struct PolyNode));
P1->link = NULL;
Attach(5, 0, &P1);
Attach(2, 1, &P1);
Attach(-3, 2, &P1);
// 新建多项式P2
P2 = (Polynomial)malloc(sizeof(struct PolyNode));
P2->link = NULL;
Attach(7, 1, &P2);
Attach(-2, 2, &P2);
Attach(4, 4, &P2);
// 输出多项式P1和P2
printf("P1: ");
PrintPoly(P1);
printf("P2: ");
PrintPoly(P2);
// 多项式相加
PP = PolyAdd(P1, P2);
// 输出相加结果
printf("P1 + P2: ");
PrintPoly(PP);
return 0;
}
```
阅读全文
相关推荐







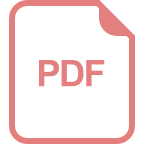
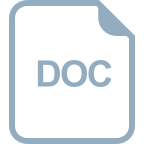
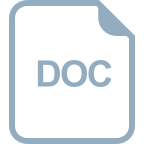
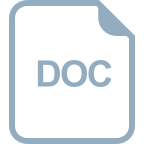




