如何用C语言实现多项式的加法运算?
时间: 2024-10-27 11:02:50 浏览: 33
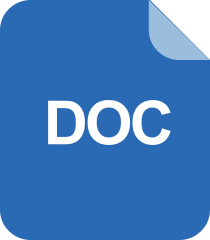
c语言多项式相加相乘
在C语言中,我们可以使用数组来表示多项式,并通过指针操作来实现多项式的加法。下面是一个简单的步骤:
1. 定义两个多项式结构体,每个包含一个系数数组和一个阶数(多项式的最高次数)。
```c
typedef struct {
int coefficients[100]; // 高次项到常数项,假设最多100次
int degree;
} Polynomial;
```
2. 创建函数来初始化多项式,给定一组系数和最高次数。
```c
void initialize_polynomial(Polynomial *poly, int* coefficients, int degree) {
for (int i = 0; i <= degree; i++) {
poly->coefficients[i] = coefficients[i];
}
poly->degree = degree;
}
```
3. 创建函数来计算两个多项式的和。遍历两个多项式的系数数组,将对应位置的系数相加。
```c
void add_polynomials(Polynomial *result, const Polynomial *poly1, const Polynomial *poly2) {
int sum_degree = poly1->degree + poly2->degree;
result->degree = sum_degree;
for (int i = 0; i <= sum_degree; i++) {
if (i < poly1->degree && i < poly2->degree)
result->coefficients[i] = poly1->coefficients[i] + poly2->coefficients[i];
else if (i >= poly1->degree)
result->coefficients[i] = poly2->coefficients[i];
else if (i >= poly2->degree)
result->coefficients[i] = poly1->coefficients[i];
}
}
```
4. 最后,你可以创建两个多项式结构体,初始化它们并调用add_polynomials函数来得到结果。
```c
int main() {
int coefficients1[] = {1, 2, 3}; // x^2 + 2x + 3
int coefficients2[] = {4, 5, 6}; // 4x^2 + 5x + 6
Polynomial poly1, poly2, result;
initialize_polynomial(&poly1, coefficients1, 2);
initialize_polynomial(&poly2, coefficients2, 2);
add_polynomials(&result, &poly1, &poly2);
// 打印结果多项式系数
for (int i = 0; i <= result.degree; i++) {
printf("%dx^%d ", result.coefficients[i], i);
}
return 0;
}
```
阅读全文
相关推荐
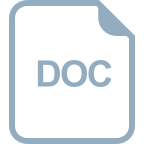
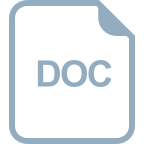




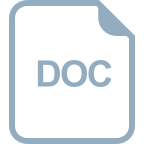
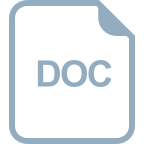


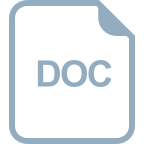




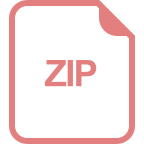
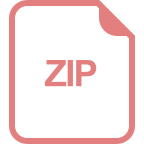
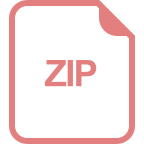