std::map<std::string, std::unique_ptr<Sensor>> _sensorMap 如何读值
时间: 2023-08-18 22:09:02 浏览: 141
要读取`_sensorMap`中的值,您可以使用`std::map`的成员函数`find`或`at`。这两个函数都可以根据给定的键来查找对应的值。
使用`find`函数的示例代码如下:
```cpp
// 假设要查找的键为key
auto iterator = _sensorMap.find(key);
if(iterator != _sensorMap.end()) {
// 找到了对应的键值对
std::unique_ptr<Sensor>& sensorPtr = iterator->second;
// 使用sensorPtr指向的Sensor对象进行操作
} else {
// 没有找到对应的键值对
}
```
使用`at`函数的示例代码如下:
```cpp
// 假设要查找的键为key
try {
std::unique_ptr<Sensor>& sensorPtr = _sensorMap.at(key);
// 使用sensorPtr指向的Sensor对象进行操作
} catch(const std::out_of_range& e) {
// 没有找到对应的键值对
}
```
需要注意的是,使用`at`函数访问不存在的键会抛出`std::out_of_range`异常,而使用`find`函数则不会抛出异常,而是返回一个指向`end()`迭代器的特殊迭代器。因此,在使用`find`函数时需要检查返回值是否等于`end()`来判断是否找到了对应的键值对。
相关问题
没有与这些操作数匹配的 "=" 运算符C/C++(349) listener_str.cpp(12, 23): 操作数类型为: std::shared_ptr<rclcpp::Subscription<std_msgs::msg::String, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<std_msgs::msg::String, std::allocator<void>>>> = std::shared_ptr<rclcpp::Subscription<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>>>>
这个错误通常是因为尝试将一个类型为`std::shared_ptr<rclcpp::Subscription<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>>>>`的变量赋值给一个类型为`std::shared_ptr<rclcpp::Subscription<std_msgs::msg::String, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<std_msgs::msg::String, std::allocator<void>>>>`的变量,这两个类型虽然都是指向`rclcpp::Subscription`的`shared_ptr`,但是模板参数不同,无法直接赋值。
解决方法是将两个类型匹配,可以通过使用`std::static_pointer_cast`将其中一个类型转换成另一个类型,例如:
```
std::shared_ptr<rclcpp::Subscription<std_msgs::msg::String, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<std_msgs::msg::String, std::allocator<void>>>> sub;
std::shared_ptr<rclcpp::Subscription<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>>>> sub_const;
// 将 sub_const 转换成 sub 的类型
sub = std::static_pointer_cast<rclcpp::Subscription<std_msgs::msg::String, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<std_msgs::msg::String, std::allocator<void>>>>(sub_const);
```
std::map<std::string, std::unique_ptr<Sensor>> _sensorMap
这是一个使用C++标准库中的`std::map`容器的示例代码,用于存储键值对,其中键是`std::string`类型,值是`std::unique_ptr<Sensor>`类型的智能指针。`Sensor`是一个用户定义的类或结构体。
通过使用`std::unique_ptr`,我们可以确保每个`Sensor`对象都具有唯一的所有权,当它们不再需要时会自动释放内存。这可以避免内存泄漏和显式的手动内存管理。
请注意,这只是代码的一部分,可能还需要其他代码来完善该类的功能,如添加、删除或查找键值对等操作。
阅读全文
相关推荐
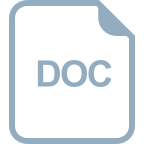
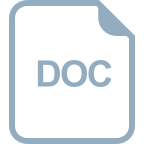
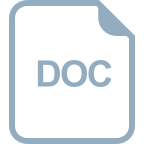













