有十个学生,每个学生的数据包括学号、姓名、3门课程的成绩,从键盘输入10个学生数据,要求输出3门课程的平均成绩,然后按照平均成绩由高到低输出各个学生的信息(包括学号、姓名、3门课程成绩、平均分数)
时间: 2024-05-28 17:07:22 浏览: 7
这是一道典型的计算机程序设计问题,需要用到结构体和排序等相关知识。以下是一个简单的C语言程序实现:
```
#include <stdio.h>
#include <string.h>
#define N 10
#define M 3
struct Student {
char id[20];
char name[20];
float score[M];
float avg_score;
};
void sort(struct Student stu[], int n);
void print(struct Student stu[], int n);
int main() {
struct Student stu[N];
float sum[M] = {0};
for (int i = 0; i < N; i++) {
printf("请输入第 %d 个学生的信息(学号 姓名 成绩1 成绩2 成绩3):\n", i+1);
scanf("%s %s %f %f %f", stu[i].id, stu[i].name, &stu[i].score, &stu[i].score, &stu[i].score);
// 计算平均分
for (int j = 0; j < M; j++) {
sum[j] += stu[i].score[j];
}
stu[i].avg_score = (stu[i].score + stu[i].score + stu[i].score) / M;
}
// 计算平均分
for (int i = 0; i < M; i++) {
sum[i] /= N;
}
printf("三门课程的平均成绩为:%.2f %.2f %.2f\n", sum, sum, sum);
// 排序并输出
sort(stu, N);
print(stu, N);
return 0;
}
void sort(struct Student stu[], int n) {
struct Student temp;
for (int i = 0; i < n-1; i++) {
for (int j = i+1; j < n; j++) {
if (stu[i].avg_score < stu[j].avg_score) {
temp = stu[i];
stu[i] = stu[j];
stu[j] = temp;
}
}
}
}
void print(struct Student stu[], int n) {
printf("按照平均成绩由高到低输出各个学生的信息:\n");
for (int i = 0; i < n; i++) {
printf("%s %s %.2f %.2f %.2f %.2f\n", stu[i].id, stu[i].name, stu[i].score, stu[i].score, stu[i].score, stu[i].avg_score);
}
}
```
相关推荐
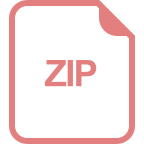
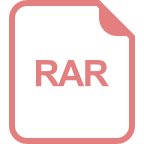
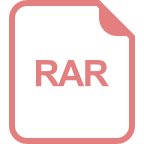













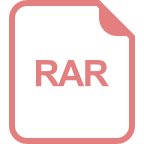