反射.netcore
时间: 2024-12-05 19:13:19 浏览: 10
反射是.NET Core中一个强大的特性,它允许程序在运行时检查和操作自身的结构和行为。通过反射,开发者可以在运行时获取类型信息、创建对象、调用方法、访问属性和字段等,而无需在编译时知道这些信息。
以下是反射在.NET Core中的一些主要用途和功能:
1. **获取类型信息**:
反射允许获取类型的元数据,如类的名称、属性、方法、事件等。
```csharp
using System;
using System.Reflection;
public class Example
{
public void DisplayTypeInfo()
{
Type type = typeof(Example);
Console.WriteLine($"Type: {type.FullName}");
PropertyInfo[] properties = type.GetProperties();
foreach (var property in properties)
{
Console.WriteLine($"Property: {property.Name}");
}
MethodInfo[] methods = type.GetMethods();
foreach (var method in methods)
{
Console.WriteLine($"Method: {method.Name}");
}
}
}
class Program
{
static void Main(string[] args)
{
Example example = new Example();
example.DisplayTypeInfo();
}
}
```
2. **创建对象**:
反射可以在运行时创建对象,而不需要在编译时知道具体的类型。
```csharp
using System;
public class Example
{
public void CreateInstance()
{
Type type = typeof(Example);
object instance = Activator.CreateInstance(type);
Console.WriteLine($"Instance created: {instance}");
}
}
class Program
{
static void Main(string[] args)
{
Example example = new Example();
example.CreateInstance();
}
}
```
3. **调用方法**:
反射可以在运行时调用对象的方法。
```csharp
using System;
public class Example
{
public void SayHello()
{
Console.WriteLine("Hello, Reflection!");
}
}
class Program
{
static void Main(string[] args)
{
Example example = new Example();
MethodInfo methodInfo = typeof(Example).GetMethod("SayHello");
methodInfo.Invoke(example, null);
}
}
```
4. **访问属性和字段**:
反射可以在运行时访问和修改对象的属性和字段。
```csharp
using System;
using System.Reflection;
public class Example
{
public string Message { get; set; }
}
class Program
{
static void Main(string[] args)
{
Example example = new Example();
Type type = typeof(Example);
PropertyInfo propertyInfo = type.GetProperty("Message");
propertyInfo.SetValue(example, "Hello, Reflection!");
Console.WriteLine(propertyInfo.GetValue(example));
}
}
```
反射是一个强大的工具,但它也有一些缺点,比如性能开销较大和安全性问题。因此,在使用反射时需要谨慎,确保在必要时才使用。
阅读全文
相关推荐
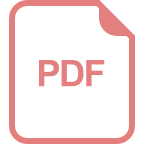
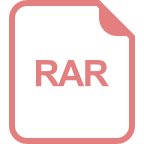
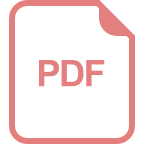
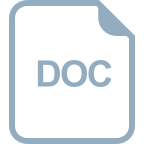
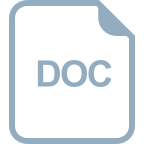

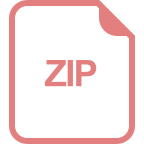
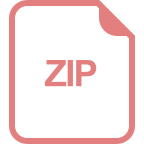
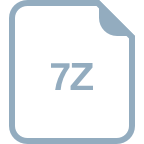
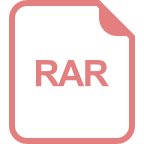
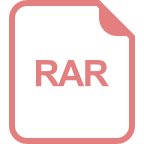
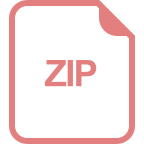
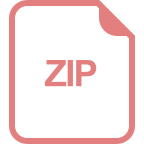
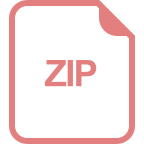
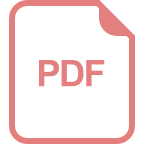
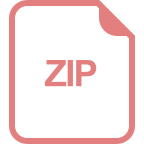
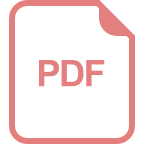
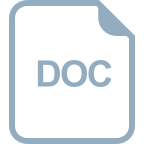
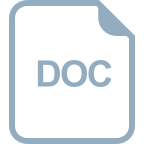