c++转为c风格字符串
时间: 2023-11-10 12:06:26 浏览: 95
要将C++字符串转换为C风格的字符串,可以使用`c_str()`函数。`c_str()`函数返回一个指向以NULL结尾的字符数组的指针,该数组包含了C++字符串的内容。
以下是一个示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string cppStr = "Hello, World!";
const char* cStr = cppStr.c_str();
std::cout << "C风格字符串: " << cStr << std::endl;
return 0;
}
```
在上述示例中,我们定义了一个C++字符串`cppStr`并将其赋值为"Hello, World!"。然后,我们使用`c_str()`函数将其转换为C风格字符串,并将结果存储在一个`const char*`类型的变量`cStr`中。最后,我们使用`std::cout`输出这个C风格字符串。
请注意,在使用`c_str()`函数转换后,C风格字符串的生命周期与原始C++字符串对象的生命周期相关联。确保在使用C风格字符串时,原始C++字符串对象仍然有效。
相关问题
将jstring转为C++字符串
在C++中,可以通过以下方式将jstring转换为C++字符串:
```c++
std::string jstring2string(JNIEnv* env, jstring jstr) {
if (jstr == NULL) {
return "";
}
const char* cstr = env->GetStringUTFChars(jstr, NULL);
std::string str(cstr);
env->ReleaseStringUTFChars(jstr, cstr);
return str;
}
```
其中,`env`是`JNIEnv*`类型的指针,表示Java Native Interface的环境指针,`jstr`是待转换的jstring对象。该函数会返回一个std::string类型的对象,表示转换后的字符串。
该函数的实现过程如下:
1. 判断jstring对象是否为空,如果为空则返回空字符串。
2. 使用`GetStringUTFChars`函数将jstring对象转换为C风格的字符串,返回指向该字符串的指针。
3. 将指针指向的字符串构造为std::string对象。
4. 使用`ReleaseStringUTFChars`函数释放GetStringUTFChars函数返回的指针。
需要注意的是,在使用`GetStringUTFChars`函数获取C风格字符串时,需要在不再使用该字符串时调用`ReleaseStringUTFChars`函数释放指针,以防止内存泄漏。
c++如何把字符串转为整数
在C++中,将字符串转换为整数可以使用标准库中的`atoi`函数,也可以使用更安全的`std::stoi`函数。
1. 使用`atoi`函数(注意:`atoi`是C语言标准库中的函数,不是C++的标准库函数,但它通常在C++环境中可用):
```cpp
#include <cstdlib> // 包含atoi函数的头文件
#include <iostream>
int main() {
const char* str = "123";
int num = atoi(str);
std::cout << num << std::endl; // 输出:123
return 0;
}
```
`atoi`函数解析C风格字符串为整数,如果转换失败,返回0。
2. 使用`std::stoi`函数:
```cpp
#include <string> // 包含std::string的头文件
#include <iostream>
int main() {
std::string str = "123";
int num = std::stoi(str);
std::cout << num << std::endl; // 输出:123
return 0;
}
```
`std::stoi`函数将字符串转换为整数。如果转换失败,它会抛出`std::invalid_argument`异常(如果字符串不包含任何数字)或`std::out_of_range`异常(如果字符串包含的数字导致整数溢出)。
使用`std::stoi`比`atoi`更安全,因为它提供了异常处理机制。
阅读全文
相关推荐
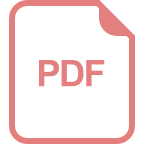
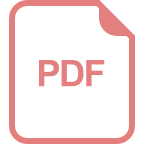
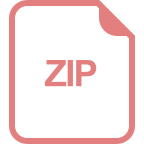
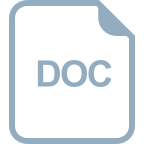
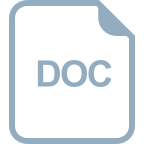
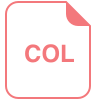
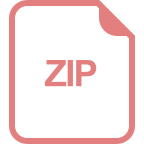
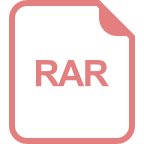

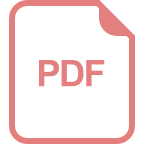
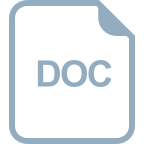
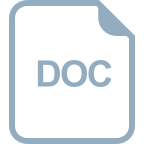



