用PYTHON 写一个电机测试数据采集分析软件 的代码
时间: 2024-10-21 17:15:53 浏览: 13
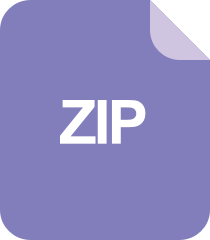
推力分析器:此代码用于基于Arduino Mega运行BLDC电机的推力分析测试台
编写一个Python电动机测试数据采集和分析软件需要涉及硬件通信、数据处理和可视化模块。这里提供一个基本框架的示例,我们假设已经有一个串口连接到电机控制器,可以接收速度和电流数据:
```python
import serial # 用于串口通信
import time # 用于时间控制
import matplotlib.pyplot as plt # 数据可视化库
class MotorDataCollector:
def __init__(self, port='COM1', baudrate=9600):
self.ser = serial.Serial(port, baudrate)
self.data_points = []
def start_data_collection(self, duration=60): # 收集60秒的数据
start_time = time.time()
while time.time() - start_time < duration:
line = self.ser.readline().decode('utf-8').strip() # 读取一行数据
if line:
speed, current = map(float, line.split(',')) # 解析数据
self.data_points.append((time.time(), speed, current))
self.stop_data_collection()
def stop_data_collection(self):
self.ser.close()
def analyze_data(self):
speed_data = [dp[1] for dp in self.data_points]
current_data = [dp[2] for dp in self.data_points]
# 可视化部分
fig, axs = plt.subplots(2, 1, sharex=True)
axs[0].plot(speed_data, label='Speed')
axs[0].set_ylabel('Speed (rpm)')
axs[1].plot(current_data, label='Current (A)')
axs[1].set_ylabel('Current (A)')
axs[1].set_xlabel('Time (s)')
axs[0].legend()
axs[1].legend()
plt.show()
if __name__ == "__main__":
collector = MotorDataCollector()
collector.start_data_collection()
collector.analyze_data()
```
在这个例子中,`MotorDataCollector`类负责串口通信和数据收集,然后将数据存储在一个列表里。`analyze_data`函数会绘制出速度和电流随时间的变化图。
阅读全文
相关推荐
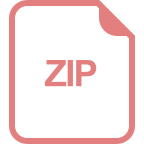
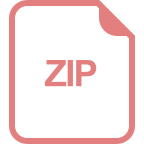
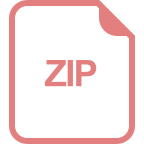
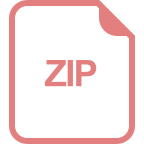
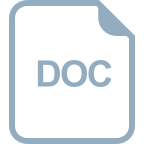
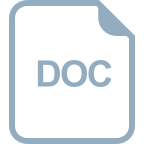
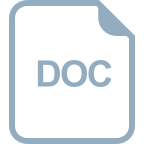
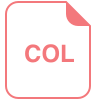
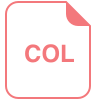
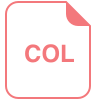
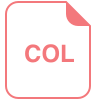
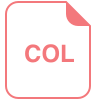
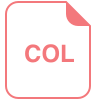
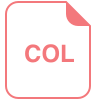
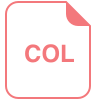
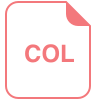
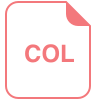