帮我用c++写一个俄罗斯方块的程序
时间: 2024-01-01 09:04:20 浏览: 33
俄罗斯方块是一款经典的游戏,使用C++编写一个俄罗斯方块的程序需要以下几个步骤:
1. 定义方块的形状和颜色,可以使用二维数组来表示方块的形状,使用颜色常量来表示方块的颜色。
2. 定义游戏区域,可以使用二维数组来表示游戏区域,每个格子可以用一个数字来表示是否有方块。
3. 定义方块的移动和旋转函数,可以使用键盘事件来控制方块的移动和旋转。
4. 定义方块的下落函数,可以使用定时器来控制方块的下落速度。
5. 定义消除行的函数,当一行被填满时,将该行消除,并将上面的方块下移一行。
6. 定义游戏结束的函数,当方块无法下落时,游戏结束。
下面是一个简单的C++俄罗斯方块程序的代码示例:
```c++
#include <iostream>
#include <conio.h>
#include <windows.h>
using namespace std;
const int WIDTH = 10;
const int HEIGHT = 20;
const int BLOCK_SIZE = 20;
const int COLORS[] = {0x000000, 0xFF0000, 0x00FF00, 0x0000FF, 0xFFFF00, 0xFF00FF, 0x00FFFF};
int board[HEIGHT][WIDTH] = {0};
int shapes[7][4][4] = {
{{0,0,0,0},
{1,1,,1},
{0,0,0,0},
{0,0,0,0}},
{{0,0,0,0},
{0,2,2,0},
{0,2,2,0},
{0,0,0,0}},
{{0,0,0,0},
{0,3,3,0},
{3,3,0,0},
{0,0,0,0}},
{{0,0,0,0},
{0,4,4,0},
{0,0,4,4},
{0,0,0,0}},
{{0,0,0,0},
{0,5,0,0},
{5,5,5,0},
{0,0,0,0}},
{{0,0,0,0},
{0,6,0,0},
{0,6,6,0},
{0,0,6,0}},
{{0,0,0,0},
{0,7,0,0},
{0,7,7,7},
{0,0,0,0}}
};
struct Point {
int x, y;
};
void drawBlock(int x, int y, int color) {
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(hConsole, color);
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
cout << " ";
}
cout << endl;
}
SetConsoleTextAttribute(hConsole, 0x0007);
}
void drawBoard() {
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] != 0) {
drawBlock(j * BLOCK_SIZE, i * BLOCK_SIZE, COLORS[board[i][j]]);
}
}
}
}
bool checkCollision(int shape[4][4], int x, int y) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shape[i][j] != 0) {
int px = x + j;
int py = y + i;
if (px < 0 || px >= WIDTH || py >= HEIGHT) {
return true;
}
if (py >= 0 && board[py][px] != 0) {
return true;
}
}
}
}
return false;
}
void placeShape(int shape[4][4], int x, int y, int color) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shape[i][j] != 0) {
int px = x + j;
int py = y + i;
board[py][px] = color;
}
}
}
}
void removeShape(int shape[4][4], int x, int y) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shape[i][j] != 0) {
int px = x + j;
int py = y + i;
board[py][px] = 0;
}
}
}
}
void rotateShape(int shape[4][4]) {
int temp[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
temp[i][j] = shape[i][j];
}
}
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
shape[i][j] = temp[3-j][i];
}
}
}
void clearLines() {
int lines = 0;
for (int i = HEIGHT-1; i >= 0; i--) {
bool full = true;
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
full = false;
break;
}
}
if (full) {
lines++;
for (int k = i; k > 0; k--) {
for (int j = 0; j < WIDTH; j++) {
board[k][j] = board[k-1][j];
}
}
for (int j = 0; j < WIDTH; j++) {
board[0][j] = 0;
}
i++;
}
}
}
bool gameOver() {
for (int j = 0; j < WIDTH; j++) {
if (board[0][j] != 0) {
return true;
}
}
return false;
}
int main() {
int shapeIndex = rand() % 7;
int shape[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
shape[i][j] = shapes[shapeIndex][i][j];
}
}
Point pos = {WIDTH/2-2, 0};
int color = shapeIndex + 1;
bool running = true;
while (running) {
if (kbhit()) {
char key = getch();
if (key == 'a' && !checkCollision(shape, pos.x-1, pos.y)) {
pos.x--;
}
if (key == 'd' && !checkCollision(shape, pos.x+1, pos.y)) {
pos.x++;
}
if (key == 's' && !checkCollision(shape, pos.x, pos.y+1)) {
pos.y++;
}
if (key == 'w') {
int temp[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
temp[i][j] = shape[i][j];
}
}
rotateShape(temp);
if (!checkCollision(temp, pos.x, pos.y)) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
shape[i][j] = temp[i][j];
}
}
}
}
if (key == 'q') {
running = false;
}
}
if (checkCollision(shape, pos.x, pos.y+1)) {
placeShape(shape, pos.x, pos.y, color);
clearLines();
if (gameOver()) {
running = false;
}
shapeIndex = rand() % 7;
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
shape[i][j] = shapes[shapeIndex][i][j];
}
}
相关推荐
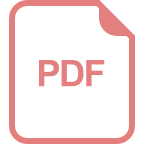









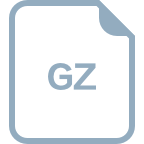
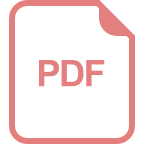