recursive_python
时间: 2024-01-11 11:55:59 浏览: 73
递归是一种在函数中调用自身的编程技巧。在Python中,你可以使用递归来解决许多问题。当涉及到处理嵌套结构的数据或者解决需要重复执行相似任务的问题时,递归是非常有用的。
以下是一个简单的示例,展示了如何使用递归函数来计算一个数的阶乘:
```python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
result = factorial(5)
print(result) # 输出: 120
```
在这个例子中,`factorial` 函数调用自身来计算给定数字 `n` 的阶乘。当 `n` 的值为 0 时,递归停止,返回 1。否则,函数将 `n` 与 `factorial(n-1)` 相乘,以得到 `n` 的阶乘。
需要注意的是,在使用递归时,确保设定一个递归终止条件,以避免无限递归。此外,递归可能会占用较多的内存和处理时间,因此在使用时要小心。
相关问题
TypeError: ForwardRef._evaluate() missing 1 required keyword-only argument: 'recursive_guard'
这个错误通常发生在Python中使用第三方库,如`dataclasses`或某些依赖于`ForwardRef`的高级数据定义时。`TypeError: ForwardRef._evaluate() missing 1 required keyword-only argument: 'recursive_guard'`意味着你在调用`_evaluate()`函数时,忘记提供名为`recursive_guard`的关键字参数。这个参数用于处理递归引用的情况。
`ForwardRef`是一个用于延迟解析类型字符串的类,在处理复杂的类结构或自嵌套的数据模型时可能会遇到这个问题。当你需要创建一个包含自身类型的实例时,如果没有正确设置`recursive_guard`,可能会导致无限循环或者其他类型的错误。
解决这个问题的方法通常是检查你的代码,确保在调用`_evaluate()`时包含了正确的参数,例如:
```python
from dataclasses import asdict, ForwardRef
ref = ForwardRef('YourRecursiveClass')
evaluated_type = ref._evaluate(recursive_guard=True)
# 现在你可以用evaluated_type来代替YourRecursiveClass
your_instance = evaluated_type()
```
python迷宫小游戏代码_python迷宫游戏,迷宫生成,解决与可视化
以下是一个简单的Python迷宫小游戏代码,包括迷宫生成、解决和可视化。
```python
import random
# 定义迷宫类
class Maze:
def __init__(self, width=10, height=10):
self.width = width
self.height = height
self.grid = self.prepare_grid()
self.configure_cells()
def prepare_grid(self):
return [[Cell(x, y) for y in range(self.height)] for x in range(self.width)]
def configure_cells(self):
for row in self.grid:
for cell in row:
x, y = cell.x, cell.y
cell.north = self.get_cell(x, y-1)
cell.east = self.get_cell(x+1, y)
cell.south = self.get_cell(x, y+1)
cell.west = self.get_cell(x-1, y)
def get_cell(self, x, y):
if x < 0 or y < 0 or x >= self.width or y >= self.height:
return None
return self.grid[x][y]
def __str__(self):
output = "+" + "---+" * self.width + "\n"
for row in self.grid:
top = "|"
bottom = "+"
for cell in row:
if cell is None:
cell = Cell(-1, -1)
body = " "
east_boundary = " " if cell.linked(cell.east) else "|"
top += body + east_boundary
south_boundary = " " if cell.linked(cell.south) else "---"
corner = "+"
bottom += south_boundary + corner
output += top + "\n"
output += bottom + "\n"
return output
# 定义细胞类
class Cell:
def __init__(self, x, y):
self.x = x
self.y = y
self.north = None
self.east = None
self.south = None
self.west = None
self.links = {}
def link(self, cell, bidi=True):
self.links[cell] = True
if bidi:
cell.link(self, False)
return self
def unlink(self, cell, bidi=True):
del self.links[cell]
if bidi:
cell.unlink(self, False)
return self
def linked(self, cell):
return cell is not None and cell in self.links
def neighbors(self):
neighbors = []
if self.north:
neighbors.append(self.north)
if self.east:
neighbors.append(self.east)
if self.south:
neighbors.append(self.south)
if self.west:
neighbors.append(self.west)
return neighbors
# 定义深度优先搜索算法
def recursive_backtracker(maze, current=None):
if current is None:
current = maze.grid[0][0]
stack = [current]
while stack:
current = stack[-1]
neighbors = [n for n in current.neighbors() if not current.linked(n)]
if not neighbors:
stack.pop()
else:
next_cell = random.choice(neighbors)
current.link(next_cell)
stack.append(next_cell)
# 定义广度优先搜索算法
def bfs(maze, start=None, end=None):
if start is None:
start = maze.grid[0][0]
if end is None:
end = maze.grid[maze.width-1][maze.height-1]
queue = [(start, [start])]
visited = set()
while queue:
(vertex, path) = queue.pop(0)
if vertex == end:
return path
if vertex not in visited:
visited.add(vertex)
for neighbor in [n for n in vertex.neighbors() if vertex.linked(n)]:
queue.append((neighbor, path + [neighbor]))
return None
# 定义主函数
def main():
maze = Maze(10, 10)
recursive_backtracker(maze)
print(maze)
path = bfs(maze)
if path:
for cell in path:
maze.grid[cell.x][cell.y] = "P"
print(maze)
if __name__ == "__main__":
main()
```
运行上面的代码会生成一个10x10的迷宫,然后使用深度优先搜索算法生成迷宫,并使用广度优先搜索算法找到从左上角到右下角的最短路径,并将路径用字母"P"标记在迷宫中。
阅读全文
相关推荐
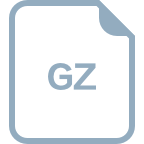
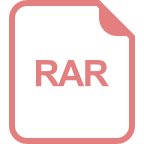
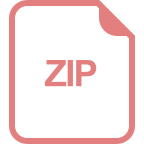
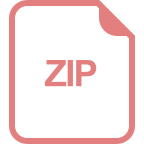
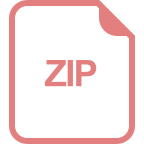
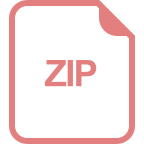
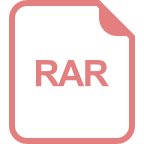
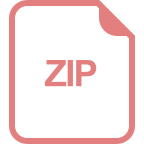
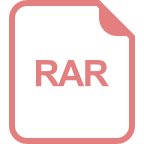
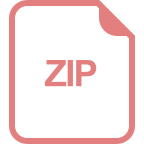
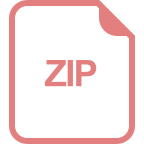


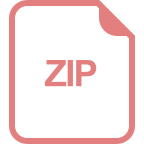
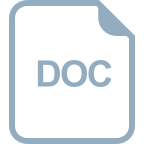
