c++双栈实现简易计算器
时间: 2023-11-22 12:06:06 浏览: 145
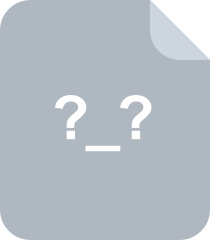
双栈实现计算器
好的,这里是C++双栈实现简易计算器的代码:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int main() {
stack<int> numStack; // 存储数字的栈
stack<char> opStack; // 存储运算符的栈
string expr; // 表达式字符串
cin >> expr;
int len = expr.length();
for (int i = 0; i < len; i++) {
char ch = expr[i];
if (ch >= '0' && ch <= '9') { // 如果是数字,直接入栈
numStack.push(ch - '0');
} else { // 如果是运算符
if (opStack.empty() || ch == '(') { // 如果运算符栈为空或者当前运算符是左括号,直接入栈
opStack.push(ch);
} else if (ch == ')') { // 如果当前运算符是右括号,弹出运算符栈中的元素直到遇到左括号
while (opStack.top() != '(') {
int num2 = numStack.top();
numStack.pop();
int num1 = numStack.top();
numStack.pop();
char op = opStack.top();
opStack.pop();
int res;
if (op == '+') {
res = num1 + num2;
} else if (op == '-') {
res = num1 - num2;
} else if (op == '*') {
res = num1 * num2;
} else {
res = num1 / num2;
}
numStack.push(res);
}
opStack.pop(); // 弹出左括号
} else { // 如果当前运算符的优先级小于等于栈顶运算符的优先级,弹出栈顶元素进行运算
while (!opStack.empty() && opStack.top() != '(' && ((ch == '*' || ch == '/') || (opStack.top() == '+' || opStack.top() == '-'))) {
int num2 = numStack.top();
numStack.pop();
int num1 = numStack.top();
numStack.pop();
char op = opStack.top();
opStack.pop();
int res;
if (op == '+') {
res = num1 + num2;
} else if (op == '-') {
res = num1 - num2;
} else if (op == '*') {
res = num1 * num2;
} else {
res = num1 / num2;
}
numStack.push(res);
}
opStack.push(ch); // 将当前运算符入栈
}
}
}
while (!opStack.empty()) { // 处理剩余的运算符
int num2 = numStack.top();
numStack.pop();
int num1 = numStack.top();
numStack.pop();
char op = opStack.top();
opStack.pop();
int res;
if (op == '+') {
res = num1 + num2;
} else if (op == '-') {
res = num1 - num2;
} else if (op == '*') {
res = num1 * num2;
} else {
res = num1 / num2;
}
numStack.push(res);
}
cout << numStack.top() << endl; // 输出最终结果
return 0;
}
```
阅读全文
相关推荐
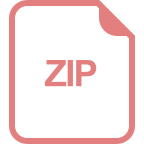
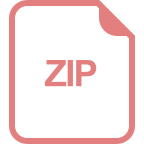
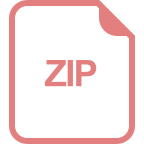
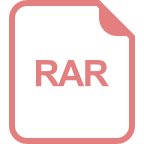
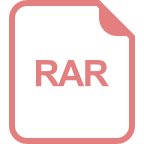
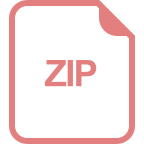
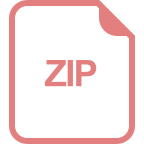
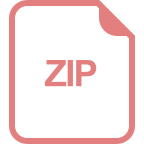
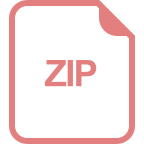
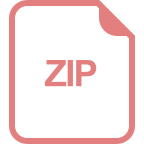
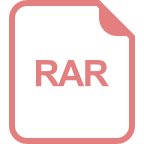
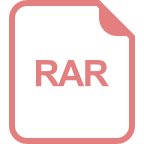
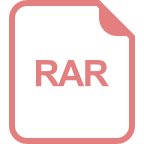
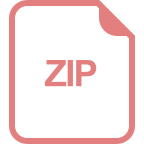
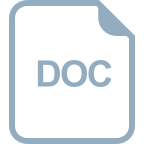