如何用C++编程来实现基于单链表的数据结构,用于计算两个集合A和B(如A={7,5,3,11}, B={2,6,3})的交集,并将结果表示为新的链表?例如,最终得到的交集链表应为{3}。
时间: 2024-10-26 19:16:30 浏览: 18
在C++中,你可以通过定义一个节点类`Node`,以及链表类`LinkedList`来实现这个功能。下面是一个简单的示例:
首先,定义节点类`Node`:
```cpp
class Node {
public:
int value;
Node* next;
Node(int value = 0, Node* next = nullptr) : value(value), next(next) {}
};
```
然后,定义链表类`LinkedList`,包括插入元素、查找元素以及获取交集链表的功能:
```cpp
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
// 插入元素
void insert(int value) {
Node* newNode = new Node(value);
if (!head) {
head = newNode;
} else {
Node* current = head;
while (current->next && current->next->value != value) {
current = current->next;
}
if (!current->next) {
current->next = newNode;
}
}
}
// 查找元素
bool contains(int value) {
Node* current = head;
while (current) {
if (current->value == value) {
return true;
}
current = current->next;
}
return false;
}
// 计算并返回交集链表
LinkedList intersect(const LinkedList& otherList) {
LinkedList intersection;
Node* ptrA = head;
Node* ptrB = otherList.head;
while (ptrA && ptrB) {
if (ptrA->value == ptrB->value) {
intersection.insert(ptrA->value); // 将交集元素添加到新链表
ptrA = ptrA->next;
ptrB = ptrB->next;
} else if (ptrA->value < ptrB->value) {
ptrA = ptrA->next;
} else {
ptrB = ptrB->next;
}
}
return intersection; // 返回交集链表
}
};
// 使用示例
LinkedList listA;
listA.insert(7);
listA.insert(5);
listA.insert(3);
listA.insert(11);
LinkedList listB;
listB.insert(2);
listB.insert(6);
listB.insert(3);
LinkedList intersection = listA.intersect(listB);
```
在这个例子中,`intersect`函数会返回一个新的链表,包含了两个输入链表A和B的公共元素(交集)。请注意,实际应用中可能需要处理内存管理,特别是当创建新链表时。
如果你想要了解更多关于链表操作或者有其他相关问题,可以继续提问:
阅读全文
相关推荐



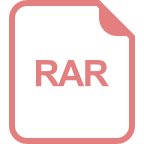
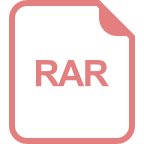


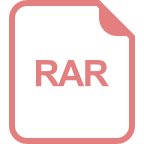
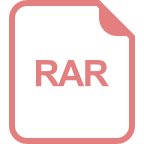
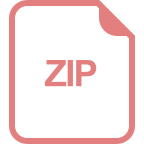



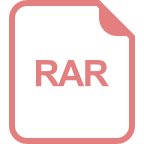




