能不能使用.net 帮我写一段图片搜索商品的代码作为参考
时间: 2024-09-18 08:12:47 浏览: 22
由于实际的代码编写涉及到许多细节和依赖项,这里提供一个简化版的C#代码片段,使用.NET的`System.Drawing`和`System.Net.Http`库作为基础,展示了基本的图片相似度比较思路。请注意,这只是一个非常初级的示例,实际应用中你需要更复杂的图像处理库(如`Emgu.CV`)和机器学习框架(如`ML.NET`):
```csharp
using System;
using System.Drawing;
using System.IO;
using System.Net.Http;
using System.Threading.Tasks;
// 假设你有一个预先训练好的图片相似度模型(例如CosineSimilarityModel)
class ImageSearchService
{
private readonly HttpClient _httpClient;
public ImageSearchService()
{
_httpClient = new HttpClient();
}
// 预测两张图片的相似度
async Task<double> CompareImagesAsync(IImageFeature image1, IImageFeature image2)
{
// 这里只是一个伪代码,真实情况应该是从模型获取预测
return await model.PredictAsync(image1.FeatureVector, image2.FeatureVector);
}
// 假设GetImageFeature是获取图片的特征函数
async Task<double> SearchProductAsync(string imageUrl, byte[] productImageBytes)
{
var productFeature = GetImageFeature(productImageBytes);
// 发送HTTP请求获取用户上传图片的特征
var userImageResponse = await _httpClient.GetAsync(imageUrl);
var userImageStream = await userImageResponse.Content.ReadAsStreamAsync();
var userImageFeature = GetImageFeature(await userImageStream.ToArray());
// 比较并返回相似度
return CompareImagesAsync(userImageFeature, productFeature).Result;
}
}
// 假设这是图片特征的简单抽象类
interface IImageFeature
{
byte[] FeatureVector { get; }
}
class ProductImageFeature : IImageFeature
{
// 实现从图片字节数组获取特征向量的方法
public byte[] FeatureVector => ...; // 获取产品图片特征的实际实现
}
// 用户上传图片的URL
string userImageUrl = "http://example.com/user-image.jpg";
byte[] productImageBytes = File.ReadAllBytes("path/to/product/image.jpg");
var service = new ImageSearchService();
double similarity = await service.SearchProductAsync(userImageUrl, productImageBytes);
```
阅读全文
相关推荐
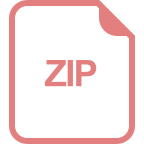
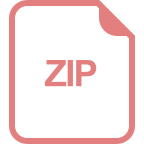
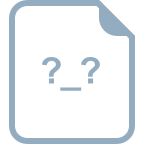
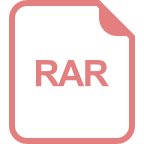
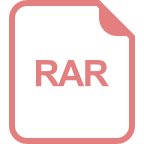
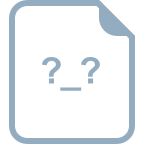
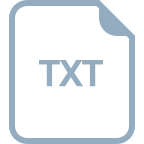
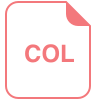


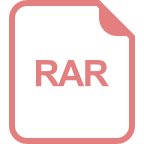
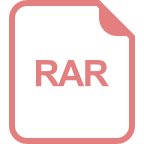
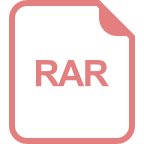
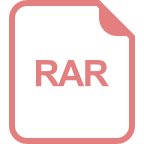
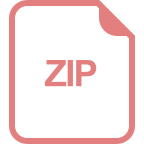
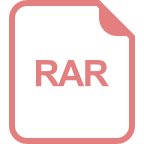
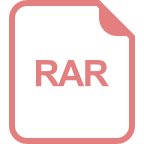
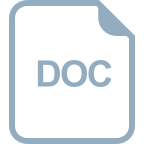