如果有括号,如何修改这段代码以正确处理?
时间: 2024-10-06 22:00:59 浏览: 16
如果有括号,我们需要对处理算法做一些调整,以便确保先计算括号内的表达式。这里可以采用一种叫做“括号优先”(Parentheses First)的方法。下面是修改后的代码示例:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <vector>
std::string precedence(char operatorSymbol) {
// 根据括号优先规则,括号的优先级最高
return operatorSymbol == '(' ? "" : "(" + precedence(operatorSymbol) + ")";
}
std::string infixToRPN(std::string exp) {
std::stack<std::pair<char, int>> st; // 用一对字符(操作符,深度)替换单个字符,记录嵌套括号的层次
std::stack<char> opStack;
std::string result;
for (size_t i = 0; i < exp.length(); ++i) {
char current = exp[i];
if (current == ' ') continue; // 忽略空格
if (isOperator(current)) {
// 检查是否有未完成的运算
while (!opStack.empty() && isOperator(opStack.top()) && precedence(opStack.top()) >= precedence(current)) {
result += opStack.top();
opStack.pop();
}
opStack.push(current); // 将当前操作符压入堆栈
} else if (current == '(') {
st.push({current, 0});
} else if (current == ')') {
// 遇到右括号,取出并计算左括号对应的运算
while (!st.empty() && st.top().first != '(') {
result += opStack.top();
opStack.pop();
st.top().second--;
if (st.top().second == 0) st.pop();
}
if (!st.empty()) st.pop(); // 出栈左括号
} else { // 当前是操作数,直接添加到结果
result += current;
}
}
// 最后处理栈顶的剩余运算符
while (!opStack.empty()) {
result += opStack.top();
opStack.pop();
}
return result;
}
int main() {
std::string infix = "A + (B * C)"; // 包含括号的中缀表达式
std::string rpn = infixToRPN(infix);
std::cout << "中缀表达式 " << infix << " 转换为后缀表达式为: " << rpn << std::endl;
return 0;
}
```
在这个修改过的版本中,我们使用了一个包含两个元素(操作符和嵌套深度)的栈,当遇到右括号时,会回溯并计算左侧括号内表达式的值。这样就可以保证括号内的运算在外部运算之前完成了。
阅读全文
相关推荐
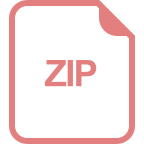
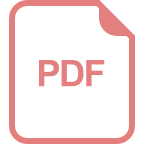
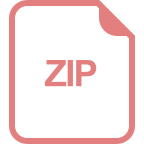






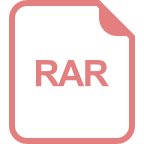
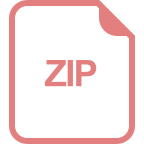







