java 实现 kmp算法
时间: 2023-08-15 17:10:09 浏览: 61
以下是Java的KMP算法实现示例:
```java
public class KMPAlgorithm {
public static int kmpSearch(String text, String pattern) {
int[] next = getNextArray(pattern);
int i = 0; // text中的指针
int j = 0; // pattern中的指针
while (i < text.length() && j < pattern.length()) {
if (j == -1 || text.charAt(i) == pattern.charAt(j)) {
i++;
j++;
} else {
j = next[j]; // 模式串向右移动
}
}
if (j == pattern.length()) {
return i - j; // 匹配成功,返回在text中的起始位置
} else {
return -1; // 匹配失败
}
}
private static int[] getNextArray(String pattern) {
int[] next = new int[pattern.length()];
next[0] = -1;
int i = 0;
int j = -1;
while (i < pattern.length() - 1) {
if (j == -1 || pattern.charAt(i) == pattern.charAt(j)) {
i++;
j++;
next[i] = j;
} else {
j = next[j];
}
}
return next;
}
public static void main(String[] args) {
String text = "ABABABCDABABCABAB";
String pattern = "ABABCABAB";
int index = kmpSearch(text, pattern);
if (index != -1) {
System.out.println("Pattern found at index " + index);
} else {
System.out.println("Pattern not found");
}
}
}
```
在上述示例中,`kmpSearch()`方法用于执行KMP算法的搜索过程,`getNextArray()`方法用于生成模式串的next数组。在`main()`方法中,我们演示了如何使用KMP算法在文本串中查找模式串的位置。
相关推荐
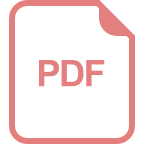














